Change Background Color of Entry Widget on Focus in Tkinter Python
In Tkinter, the Entry
widget is used to take user input. You can change the background color of an Entry widget when it gains or loses focus using the bind()
method.
In this tutorial, we will demonstrate different ways to change the background color of an Entry widget on focus in a Tkinter Entry widget.
Examples
1. Changing Background Color of Entry Widget on Focus and Blur
In this example, we will change the background color of an Entry widget to light yellow when it gains focus and revert to white when it loses focus.
import tkinter as tk
root = tk.Tk()
root.title("Change Entry BG on Focus - tutorialkart.com")
root.geometry("400x200")
def on_focus(event):
event.widget.config(bg="lightyellow")
def on_blur(event):
event.widget.config(bg="white")
entry = tk.Entry(root, font=("Arial", 14))
entry.pack(pady=20, padx=20, ipadx=10, ipady=5)
entry.bind("<FocusIn>", on_focus)
entry.bind("<FocusOut>", on_blur)
root.mainloop()
Output in Windows:
When Entry widget is out of focus.
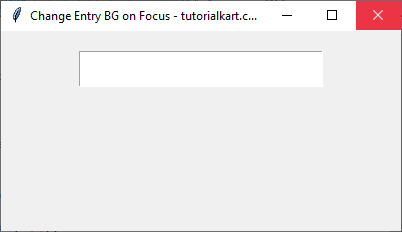
Click on the Entry widget to get focus, and notice the change in background color.
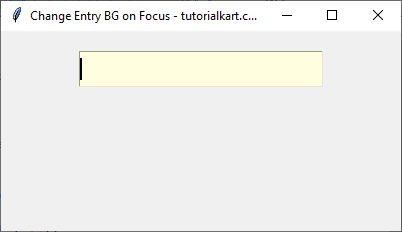
2. Changing Background Color of Entry Widget with Multiple Entry Fields
In this example, we apply the same focus and blur effect to multiple Entry fields.
import tkinter as tk
root = tk.Tk()
root.title("Entry BG Change on Focus - tutorialkart.com")
root.geometry("400x200")
def on_focus(event):
event.widget.config(bg="lightblue")
def on_blur(event):
event.widget.config(bg="white")
entry1 = tk.Entry(root, font=("Arial", 14))
entry1.pack(pady=10, padx=20, ipadx=10, ipady=5)
entry1.bind("<FocusIn>", on_focus)
entry1.bind("<FocusOut>", on_blur)
entry2 = tk.Entry(root, font=("Arial", 14))
entry2.pack(pady=10, padx=20, ipadx=10, ipady=5)
entry2.bind("<FocusIn>", on_focus)
entry2.bind("<FocusOut>", on_blur)
root.mainloop()
Output in Windows:
When no Entry widget is in focus.
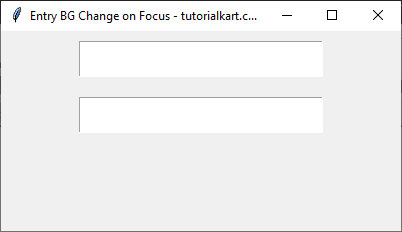
When first Entry widget is in focus.
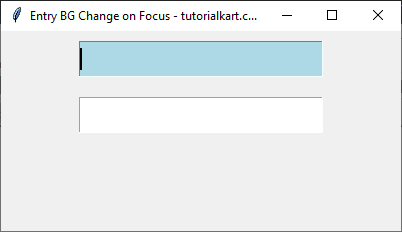
When second Entry widget is in focus.
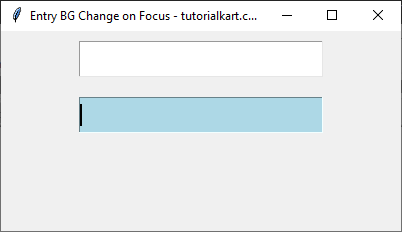
Conclusion
In this tutorial, we explored different methods to change the background color of an Entry widget when it gains or loses focus. The bind()
method was used to detect focus events and dynamically change the background color.