Change Background Color of Entry Widget on Hover in Tkinter Python
In Tkinter, the Entry
widget allows users to enter text. You can change its background color dynamically when the mouse hovers over it using the bind()
method. This technique improves the user experience by providing a visual cue when the Entry field is active.
In this tutorial, we will explore multiple methods to change the background color of the Entry widget when the user hovers over it in a Tkinter Entry widget.
Examples
1. Changing Background Color of Entry Widget on Hover Using bind()
In this example, we use the bind()
method to change the background color when the mouse enters and leaves the Entry widget.
import tkinter as tk
# Create main application window
root = tk.Tk()
root.title("Entry Hover Example - tutorialkart.com")
root.geometry("400x200")
# Function to change background color on hover
def on_enter(event):
entry.config(bg="lightblue")
def on_leave(event):
entry.config(bg="white")
# Create an Entry widget
entry = tk.Entry(root, font=("Arial", 14))
entry.pack(pady=20, padx=20)
# Bind hover events
entry.bind("<Enter>", on_enter)
entry.bind("<Leave>", on_leave)
# Run the Tkinter event loop
root.mainloop()
Output in Windows:
The Entry field turns light blue when hovered over and reverts to white when the mouse leaves.
Initial look of the Entry Widget.
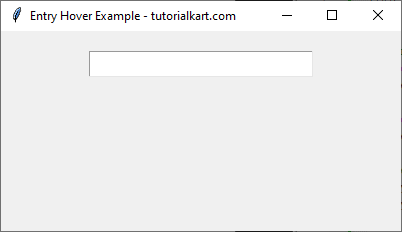
When hovered over the Entry Widget.
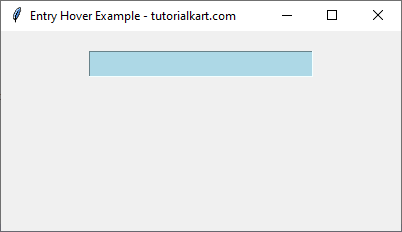
2. Changing Background Color with Multiple Entry Widgets
In this example, we apply the hover effect to multiple Entry fields.
import tkinter as tk
root = tk.Tk()
root.title("Multiple Entry Hover Example - tutorialkart.com")
root.geometry("400x200")
def on_enter(event):
event.widget.config(bg="green")
def on_leave(event):
event.widget.config(bg="white")
# Create multiple Entry widgets
entries = []
for i in range(3):
entry = tk.Entry(root, font=("Arial", 14))
entry.pack(pady=5, padx=20)
entry.bind("<Enter>", on_enter)
entry.bind("<Leave>", on_leave)
entries.append(entry)
root.mainloop()
Output in Windows:
Each Entry field changes to green when hovered over and reverts to white when the mouse leaves.
The following is the screenshot when mouse hovered on the third Entry widget.
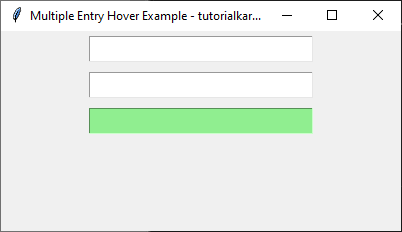
3. Changing Background and Border Color of Entry Widget on Hover
We can also change both the background color and border color (highlight color) when the user hovers over the Entry widget.
import tkinter as tk
root = tk.Tk()
root.title("Entry Hover with Border - tutorialkart.com")
root.geometry("400x200")
def on_enter(event):
event.widget.config(bg="lightyellow", highlightbackground="orange", highlightthickness=2)
def on_leave(event):
event.widget.config(bg="white", highlightbackground="gray", highlightthickness=1)
# Create an Entry widget
entry = tk.Entry(root, font=("Arial", 14), highlightthickness=1, highlightbackground="gray")
entry.pack(pady=20, padx=20)
entry.bind("<Enter>", on_enter)
entry.bind("<Leave>", on_leave)
root.mainloop()
Output in Windows:
The Entry field turns light yellow and the border changes to orange when hovered over. It reverts to white with a gray border when the mouse leaves.
Initial look of the Entry widget.
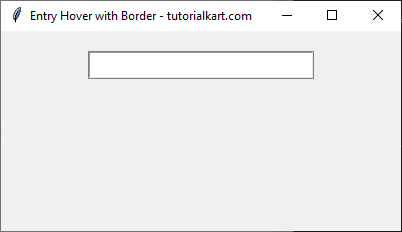
After hovering on the Entry widget.
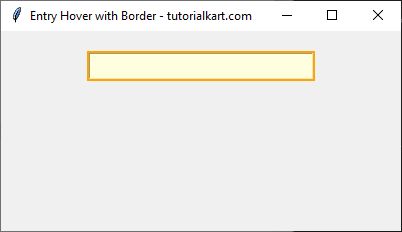
Conclusion
In this tutorial, we explored how to change the background color of a Tkinter Entry
widget on hover. We used the bind()
method to apply color changes dynamically.
- The first example demonstrated how to change the background color of a single Entry widget.
- The second example applied the hover effect to multiple Entry widgets.
- The third example modified both the background and border color for better visibility.