Change Background Color of Text Widget on Focus in Tkinter Python
In Tkinter, you can change the background color of a Text
widget when it gains focus using the focusin
event and restore its original background color when it loses focus using the focusout
event.
We achieve this by binding event listeners to the Text
widget. When the widget gains focus, we modify its bg
property to a different color, and when it loses focus, we restore the default background color.
In this tutorial, we will go through different examples demonstrating how to change the background color of a Tkinter Text widget on focus.
Examples
1. Changing Background Color of Text Widget on Focus and Losing Focus
In this example, we will create a Tkinter window with a Text
widget whose background color changes to yellow when it gains focus and returns to white when it loses focus.
We are using the bind
method to associate the <FocusIn>
and <FocusOut>
events with functions that modify the bg
property of the Text
widget. The on_focus_in
function changes the background to yellow, and the on_focus_out
function restores it to white.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Text Focus Background - tutorialkart.com")
root.geometry("400x200")
# Function to change background color when focused
def on_focus_in(event):
text_widget.config(bg="yellow")
# Function to reset background color when focus is lost
def on_focus_out(event):
text_widget.config(bg="white")
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=20)
# Bind focus events
text_widget.bind("<FocusIn>", on_focus_in)
text_widget.bind("<FocusOut>", on_focus_out)
root.mainloop()
Output in Windows:
The following screen appears when you run the program.
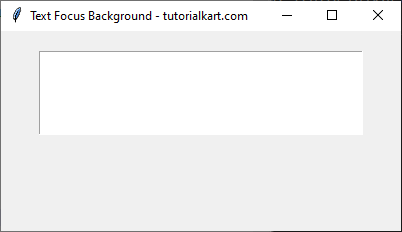
When the text widget is clicked and focused, its background turns yellow. Let us click on the Text widget.
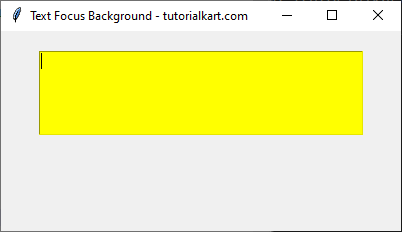
The background turned yellow.
When the user clicks elsewhere, the background resets to white.
2. Changing Background Color and Text Color for Text Widget on Focus
In this example, we will modify both the background color and the text color when the Text widget gains focus. The background changes to light blue, and the text color changes to dark blue.
We define on_focus_in
to set the background to light blue and text color to dark blue, while on_focus_out
resets them to their default values. The bind
method is used to associate these functions with the <FocusIn>
and <FocusOut>
events.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Text Focus Color Change - tutorialkart.com")
root.geometry("400x200")
# Function to change background and text color when focused
def on_focus_in(event):
text_widget.config(bg="lightblue", fg="darkblue")
# Function to reset background and text color when focus is lost
def on_focus_out(event):
text_widget.config(bg="white", fg="black")
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=20)
# Bind focus events
text_widget.bind("<FocusIn>", on_focus_in)
text_widget.bind("<FocusOut>", on_focus_out)
root.mainloop()
Output in Windows:
When the text widget is clicked and focused, its background turns light blue, and text color turns dark blue.
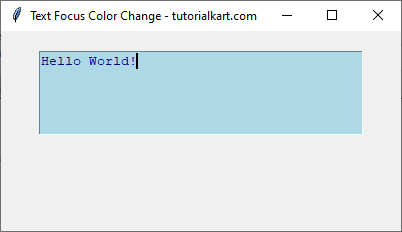
When the user clicks outside, the background resets to white, and the text color resets to black.
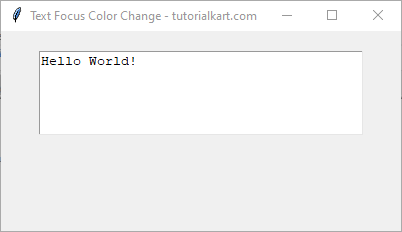
Conclusion
In this tutorial, we explored how to change the background color of a Tkinter Text
widget when it gains and loses focus. This is achieved using the focusin
and focusout
events bound to functions that modify the bg
property.
- Example 1 demonstrated changing only the background color.
- Example 2 extended it by also changing the text color on focus.