Change Background Color of Text Widget on Hover
In Tkinter, you can change the background color of a Text
widget when the user hovers over it using the bind()
function. This method allows you to trigger an event when the mouse enters or leaves the widget, dynamically updating its appearance.
To achieve this, we use the bind()
method to attach event listeners to the Text
widget, detecting when the cursor enters (<Enter>
) and leaves (<Leave>
) the widget. Inside the event-handling functions, we update the background color using the config()
method.
In this tutorial, we will go through multiple examples demonstrating how to change the background color of a Tkinter Text
widget on hover.
Examples
1. Changing Background Color of Text Widget on Hover
In this example, we will create a Tkinter Text
widget that changes its background color when the mouse enters and reverts when the mouse leaves.
We define two functions: on_hover()
and on_leave()
. The on_hover()
function sets the background color to light yellow, while on_leave()
restores the original white background. We bind these functions to the <Enter>
and <Leave>
events using the bind()
method.
main.py
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Text Hover Example - tutorialkart.com")
root.geometry("400x200")
# Function to change background color on hover
def on_hover(event):
text_widget.config(bg="lightyellow")
# Function to revert background color on mouse leave
def on_leave(event):
text_widget.config(bg="white")
# Create Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=20)
# Bind hover events
text_widget.bind("<Enter>", on_hover)
text_widget.bind("<Leave>", on_leave)
# Run the main event loop
root.mainloop()
Output in Windows:
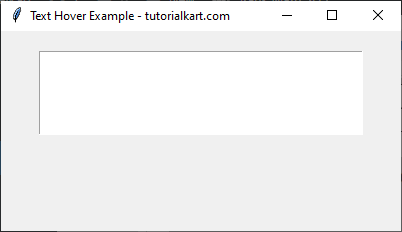
When the mouse enters the Text
widget, its background color changes to light yellow.
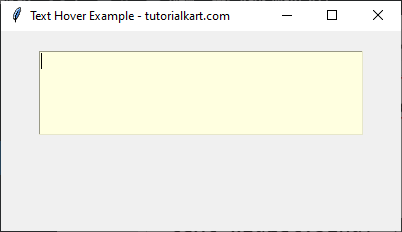
When the mouse leaves, it returns to white.
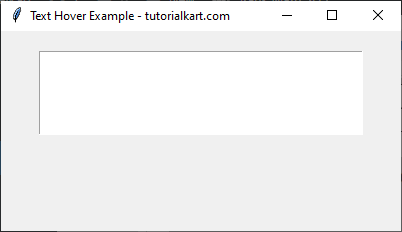
2. Changing Background and Foreground Colors of Text Widget on Hover
In this example, we enhance the previous functionality by changing both the background and text color on hover.
We define on_hover()
to set the background to light blue and the foreground (text color) to dark blue. The on_leave()
function restores the default colors. We bind these functions to <Enter>
and <Leave>
events, ensuring the color changes happen dynamically.
main.py
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Text Hover Color Change - tutorialkart.com")
root.geometry("400x200")
# Function to change background and foreground color on hover
def on_hover(event):
text_widget.config(bg="lightblue", fg="darkblue")
# Function to revert colors on mouse leave
def on_leave(event):
text_widget.config(bg="white", fg="black")
# Create Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=20)
# Bind hover events
text_widget.bind("<Enter>", on_hover)
text_widget.bind("<Leave>", on_leave)
# Run the main event loop
root.mainloop()
Output in Windows:
When the window appears, enter some value in the text widget.
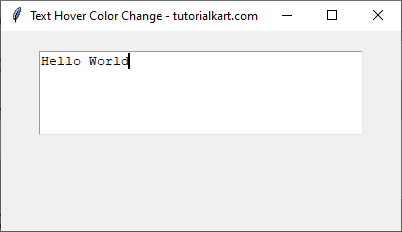
When the mouse enters the Text
widget, its background color turns light blue, and the text color changes to dark blue.
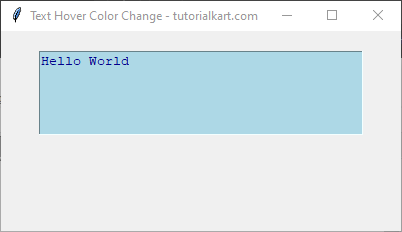
On mouse leave, the colors revert to white and black, respectively.
Conclusion
In this tutorial, we explored how to use the bind()
method in Tkinter to dynamically change the background and foreground color of a Text
widget when the mouse hovers over it.
- We used the
<Enter>
event to detect when the cursor enters the widget. - The
<Leave>
event restores the original colors when the mouse exits. - The
config()
method was used to change the colors dynamically.