Change Button Color after Clicking in Tkinter Python
In Tkinter, you can change the color of a Button after it is clicked using the config()
method or the configure()
method. This allows dynamic changes to the button’s properties like background color (bg
) and text color (fg
).
In this tutorial, we will explore different ways to change a button’s color upon clicking in a Tkinter Button.
Examples
1. Change Button Background Color on Click
In this example, clicking the button changes its background color from light gray to yellow.
import tkinter as tk
root = tk.Tk()
root.title("Change Button Color - tutorialkart.com")
root.geometry("400x200")
def change_color():
button.config(bg="yellow") # Change background color
# Create a button
button = tk.Button(root, text="Click Me", bg="lightgray", command=change_color)
button.pack(pady=20)
root.mainloop()
Output:
A button appears with a light gray background.
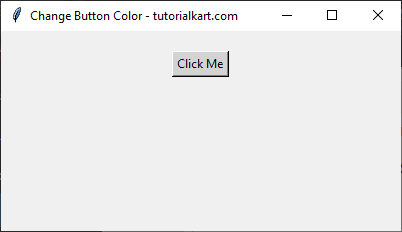
Clicking it changes its background to yellow.
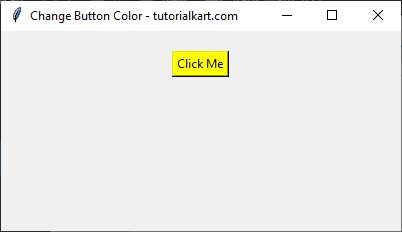
2. Change Button Text Color on Click
This example changes the button’s text color (fg
) from black to white after clicking.
import tkinter as tk
root = tk.Tk()
root.title("Change Button Text Color - tutorialkart.com")
root.geometry("400x200")
def change_text_color():
button.config(fg="white") # Change text color
# Create a button
button = tk.Button(root, text="Click Me", fg="black", bg="red", command=change_text_color)
button.pack(pady=20)
root.mainloop()
Output:
A button with black text appears.
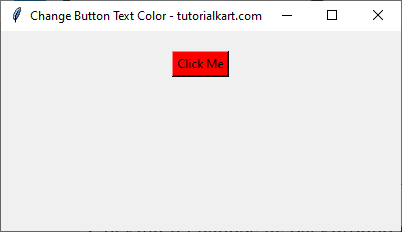
Clicking it changes the text color to white.
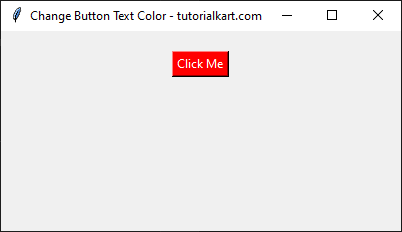
3. Toggle Button Color on Each Click
This example alternates the button’s background color between green and red with each click.
import tkinter as tk
root = tk.Tk()
root.title("Toggle Button Color - tutorialkart.com")
root.geometry("400x200")
def toggle_color():
current_color = button.cget("bg")
new_color = "green" if current_color == "red" else "red"
button.config(bg=new_color)
# Create a button
button = tk.Button(root, text="Toggle Color", bg="red", command=toggle_color)
button.pack(pady=20)
root.mainloop()
Output:
The button starts with a red background.
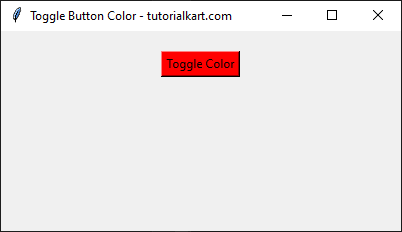
Clicking it alternates the color between red and green.
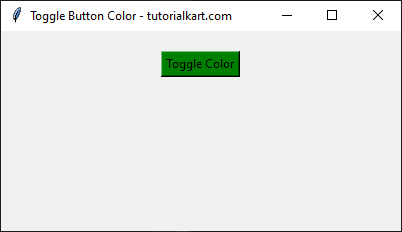
Click on the button again.
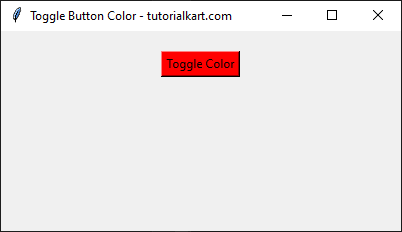
4. Change Button Color Using a Random Color
In this example, the button’s background color changes to a random color on each click.
import tkinter as tk
import random
root = tk.Tk()
root.title("Random Button Color - tutorialkart.com")
root.geometry("400x200")
def change_random_color():
colors = ["red", "blue", "green", "yellow", "purple", "orange"]
button.config(bg=random.choice(colors))
# Create a button
button = tk.Button(root, text="Random Color", bg="gray", command=change_random_color)
button.pack(pady=20)
root.mainloop()
Output:
Clicking the button changes its background color to a random color from the list.
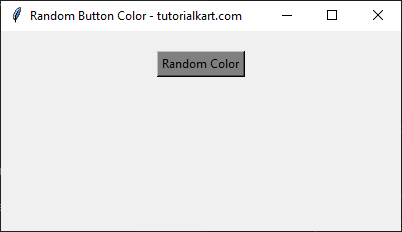
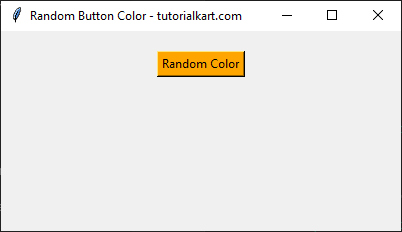
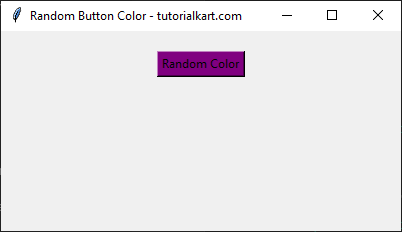
Conclusion
In this tutorial, we explored different ways to change a button’s color after clicking in Tkinter:
- Changing the background color using
bg
- Changing the text color using
fg
- Toggling colors on each click
- Setting a random color on click
By using the config()
method, you can dynamically update button properties and improve user interaction in your Tkinter applications.