Change Button Color on Hovering in Tkinter Python
In Tkinter, you can change the button color when the mouse hovers over it using the bind()
method. The bind()
method allows you to attach event handlers to a widget. In this case, we use <Enter>
for mouse hover and <Leave>
for when the mouse moves away.
In this tutorial, we will explore different examples to modify a button’s background color dynamically when the user hovers over it.
Examples
1. Changing Background Color on Hover
In this example, we will change the button background color when the user hovers over it and revert back when the mouse moves away.
import tkinter as tk
root = tk.Tk()
root.title("Button Hover Effect - tutorialkart.com")
root.geometry("400x200")
def on_enter(event):
button.config(bg="orange")
def on_leave(event):
button.config(bg="white")
# Create a button
button = tk.Button(root, text="Hover Over Me", bg="white", font=("Arial", 12))
button.pack(pady=20)
# Bind hover events
button.bind("<Enter>", on_enter)
button.bind("<Leave>", on_leave)
root.mainloop()
Output:
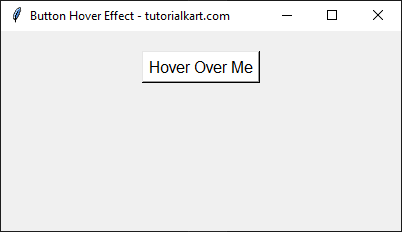
The button turns light orange when hovered.
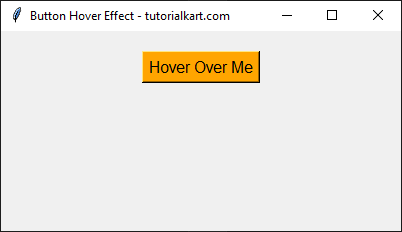
And returns to white when the mouse moves away.
2. Changing Text and Background Color on Hover
In this example, we will modify both the text color and background color on hovering.
import tkinter as tk
root = tk.Tk()
root.title("Button Hover Effect - tutorialkart.com")
root.geometry("400x200")
def on_enter(event):
button.config(bg="black", fg="white")
def on_leave(event):
button.config(bg="white", fg="black")
# Create a button
button = tk.Button(root, text="Hover Over Me", bg="white", fg="black", font=("Arial", 12))
button.pack(pady=20)
# Bind hover events
button.bind("<Enter>", on_enter)
button.bind("<Leave>", on_leave)
root.mainloop()
Output:
The button changes to black with white text when hovered and returns to white with black text when the mouse moves away.
On hovering the button:
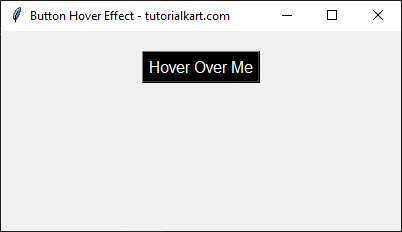
On leaving the button:
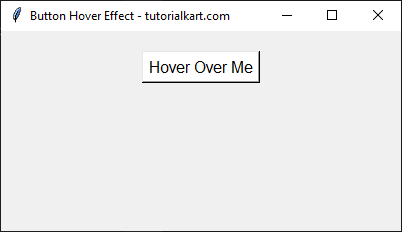
Conclusion
In this tutorial, we learned how to change a button’s color dynamically in Tkinter when the user hovers over it. We explored:
- Changing the button background color on hover.
- Changing both text and background color on hover.
These techniques allow for interactive and visually appealing buttons in Tkinter applications.