Change Text Color for Label on Hovering in Tkinter Python
In Tkinter, you can change the text color of a Label widget dynamically when the mouse hovers over it. This is achieved by binding the <Enter>
and <Leave>
events to functions that update the foreground color of the Label.
In this tutorial, we will go through different examples to change the text color of a Label widget when the user hovers over it in a Tkinter Label.
Examples
1. Change Text Color on Hover
In this example, we will change the text color of a Label when the mouse hovers over it.
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com")
root.geometry("400x200")
def on_enter(e):
label.config(fg="red") # Change text color to red
def on_leave(e):
label.config(fg="black") # Change text color back to black
# Create a Label
label = tk.Label(root, text="Hover over me!", font=("Arial", 14))
label.pack(pady=20)
# Bind hover events
label.bind("<Enter>", on_enter)
label.bind("<Leave>", on_leave)
root.mainloop()
Output in Windows:
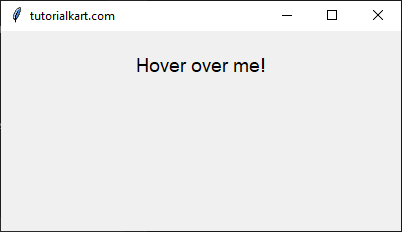
Hovering on the label widget changes the font color (foreground color) to red.
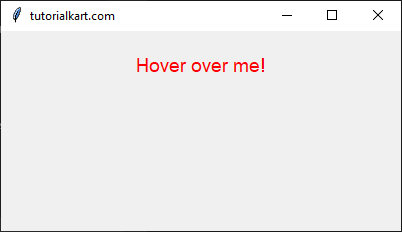
2. Change Text Color and Background on Hover
In this example, we change both the text color and the background color when hovering over the Label.
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com")
root.geometry("400x200")
def on_enter(e):
label.config(fg="white", bg="blue") # Change text to white and background to blue
def on_leave(e):
label.config(fg="black", bg="white") # Reset colors
# Create a Label
label = tk.Label(root, text="Hover over me!", font=("Arial", 14), bg="white", fg="black")
label.pack(pady=20)
# Bind hover events
label.bind("<Enter>", on_enter)
label.bind("<Leave>", on_leave)
root.mainloop()
Output in Windows:
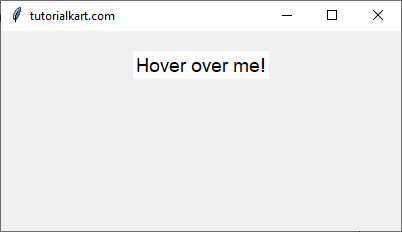
Hovering on the label changes the background color and the text color.
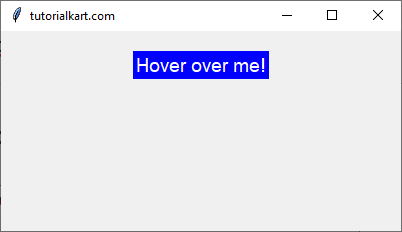
3. Change Text Color for Multiple Labels on Hover
In this example, we apply the hover effect to multiple labels by using a loop.
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com")
root.geometry("400x200")
def on_enter(e):
e.widget.config(fg="blue") # Change text color to blue
def on_leave(e):
e.widget.config(fg="black") # Reset text color
# Create multiple labels
labels = [
tk.Label(root, text="Label 1", font=("Arial", 14)),
tk.Label(root, text="Label 2", font=("Arial", 14)),
tk.Label(root, text="Label 3", font=("Arial", 14)),
]
# Pack and bind hover events
for label in labels:
label.pack(pady=5)
label.bind("<Enter>", on_enter)
label.bind("<Leave>", on_leave)
root.mainloop()
Conclusion
In this tutorial, we explored different ways to change the text color of a Label widget in Tkinter when hovered over. We covered:
- Changing only the text color
- Changing both the text color and background color
- Applying hover effects to multiple labels using loops
By using the <Enter>
and <Leave>
events, you can create interactive UI elements that enhance user experience in your Tkinter applications.