Clear Entry Widget on Button Click in Tkinter Python
In Tkinter, the Entry
widget is used to accept user input. Sometimes, we may need to clear the input field when a button is clicked. This can be done using the delete()
method of the Entry widget.
The syntax for clearing the Entry field is:
entry_widget.delete(0, tk.END)
Here, 0
represents the starting index, and tk.END
represents the end of the input field.
Examples
1. Clear Entry Field on Button Click
In this example, we create a Tkinter window with an Entry widget and a Button. When the button is clicked, the input field is cleared.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Clear Entry Example - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget
entry = tk.Entry(root, width=30)
entry.pack(pady=20)
# Function to clear the entry field
def clear_entry():
entry.delete(0, tk.END)
# Create a button to clear the Entry field
clear_button = tk.Button(root, text="Clear", command=clear_entry)
clear_button.pack()
# Run the application
root.mainloop()
Output in Windows:
Enter some value in the Entry widget.
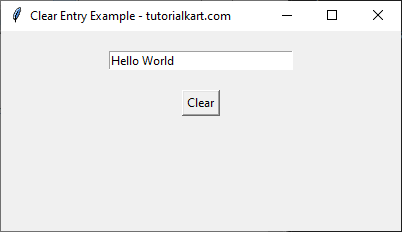
Now, click on the Clear button.
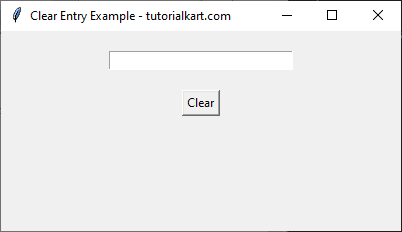
The Entry widget is cleared.
2. Clear Entry Field on Button Click with Default Text
In this example, the Entry widget contains a default text value. When the button is clicked, the text field is cleared.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Clear Entry Example - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget with default text
entry = tk.Entry(root, width=30)
entry.insert(0, "Enter your name")
entry.pack(pady=20)
# Function to clear the entry field
def clear_entry():
entry.delete(0, tk.END)
# Create a button to clear the Entry field
clear_button = tk.Button(root, text="Clear", command=clear_entry)
clear_button.pack()
# Run the application
root.mainloop()
Output in Windows:
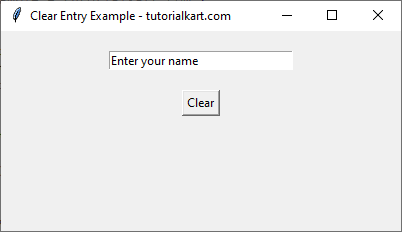
Click on the Clear button.
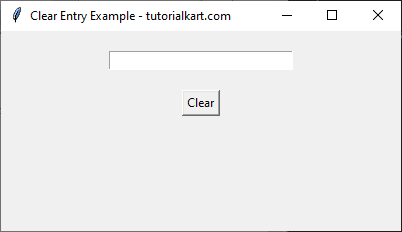
3. Clear Entry Field and Display Message
In this example, after clearing the Entry field, a message is displayed on a Label widget to notify the user.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Clear Entry Example - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget
entry = tk.Entry(root, width=30)
entry.pack(pady=10)
# Create a label for displaying messages
message_label = tk.Label(root, text="", fg="green")
message_label.pack()
# Function to clear the entry field and display a message
def clear_entry():
entry.delete(0, tk.END)
message_label.config(text="Entry field cleared!")
# Create a button to clear the Entry field
clear_button = tk.Button(root, text="Clear", command=clear_entry)
clear_button.pack()
# Run the application
root.mainloop()
Output in Windows:
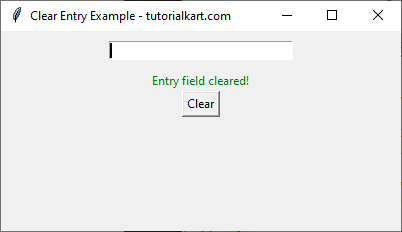
Conclusion
In this tutorial, we explored how to clear the text inside an Entry widget on button click in Tkinter. The delete(0, tk.END)
method is used to remove all content from the Entry field. Additionally, we covered different use cases, such as clearing fields with default text and displaying a message after clearing the field.