Clear Text Widget on Button Click in Tkinter Python
In Tkinter, the Text
widget is used to create multi-line text input fields. Sometimes, we need to clear its contents when a button is clicked. We can do this by using the delete()
method, which removes text from the widget.
The syntax for clearing the text widget is:
text_widget.delete("1.0", tk.END)
In this tutorial, we will demonstrate how to clear the Text
widget using a Button in Tkinter Python.
Examples
1. Clearing a Text Widget on Button Click
In this example, we will create a Tkinter window containing a Text widget and a Button. Clicking the button will clear all the text inside the Text widget.
We start by creating the main window using tk.Tk()
and setting its title to “tutorialkart.com”. The Text
widget is placed inside the window, allowing multi-line input. We define a function clear_text()
, which uses text_widget.delete("1.0", tk.END)
to remove all text when the button is clicked. The button is then assigned this function via the command
parameter.
main.py
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("tutorialkart.com")
root.geometry("400x200")
# Function to clear the Text widget
def clear_text():
text_widget.delete("1.0", tk.END)
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Create a Button to clear the text
clear_button = tk.Button(root, text="Clear Text", command=clear_text)
clear_button.pack(pady=5)
# Run the application
root.mainloop()
Output in Windows:
A window appears with a multi-line text input box and a “Clear Text” button.
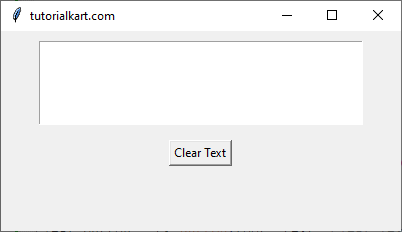
Let us enter some text, Hello World.
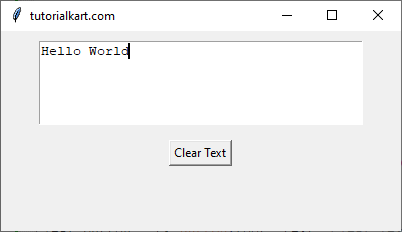
When the button is clicked, all text inside the Text widget is removed.
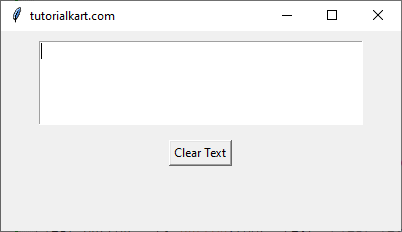
2. Clearing Text After User Confirmation
In this example, we add a confirmation step before clearing the text. When the button is clicked, a message box appears, asking the user for confirmation.
We define a function confirm_clear()
, which calls messagebox.askyesno()
to prompt the user. If the user selects “Yes”, the clear_text()
function is executed, which clears the text using text_widget.delete("1.0", tk.END)
. This ensures that accidental text removal is prevented.
main.py
import tkinter as tk
from tkinter import messagebox
# Create main window
root = tk.Tk()
root.title("tutorialkart.com")
root.geometry("400x200")
# Function to clear the Text widget with confirmation
def confirm_clear():
if messagebox.askyesno("Confirm", "Are you sure you want to clear the text?"):
clear_text()
def clear_text():
text_widget.delete("1.0", tk.END)
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Create a Button to clear the text with confirmation
clear_button = tk.Button(root, text="Clear Text", command=confirm_clear)
clear_button.pack(pady=5)
# Run the application
root.mainloop()
Output in Windows:
A window appears with a multi-line text input box and a “Clear Text” button.
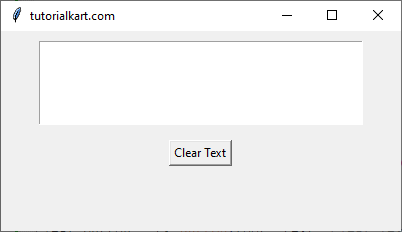
You may enter some text.
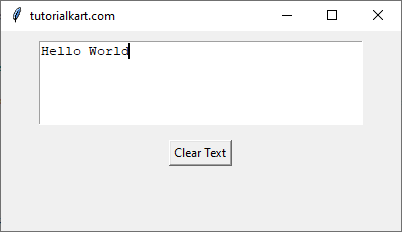
When the button is clicked, a confirmation dialog appears.
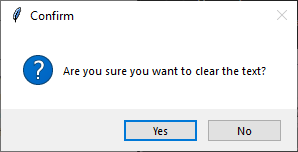
If the user selects “Yes,” the text is cleared; otherwise, it remains unchanged. Let us click on Yes button.
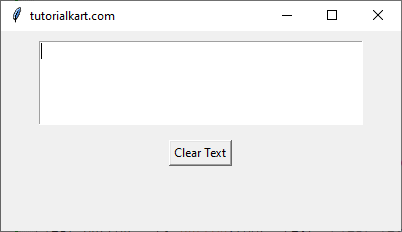
Conclusion
In this tutorial, we explored how to clear a Text
widget using a button click in Tkinter Python. We used the delete()
method to remove text and also implemented a confirmation step using messagebox.askyesno()
. These methods help control user input effectively in Tkinter applications.