Delete Last Character in Text Widget in Tkinter Python
In Tkinter, the Text
widget is used to allow multiline text input. Sometimes, you may need to delete the last character entered in the Text widget dynamically. This can be done using the delete()
method of the Text widget.
The syntax to remove the last character from a Text widget is:
text_widget.delete("end-2c")
In this tutorial, we will go through different examples to remove the last character from a Tkinter Text
widget in a Tkinter application.
Examples
1. Deleting the Last Character Using a Button
In this example, we create a Tkinter window with a Text
widget where the user can enter text. A button labeled Delete Last Character
is provided to remove the last character from the text field.
When the button is clicked, the function delete_last_character()
is triggered. This function uses text_widget.delete("end-2c")
to remove the last character. The end-2c
index tells Tkinter to delete the character before the cursor position.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Delete Last Character - tutorialkart.com")
root.geometry("400x200")
# Function to delete the last character
def delete_last_character():
text_widget.delete("end-2c") # Deletes the last character
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Create a Button to delete last character
delete_button = tk.Button(root, text="Delete Last Character", command=delete_last_character)
delete_button.pack(pady=10)
# Run the main event loop
root.mainloop()
Output in Windows:
A Text widget appears where the user can type text.
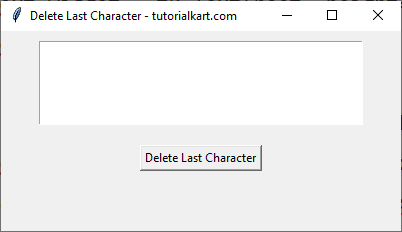
Type some text into it.
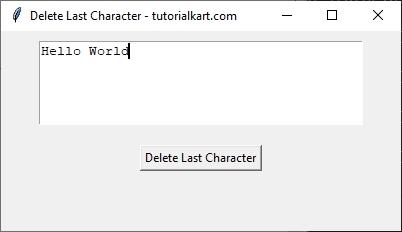
Clicking the “Delete Last Character” button removes the last character from the text entered.
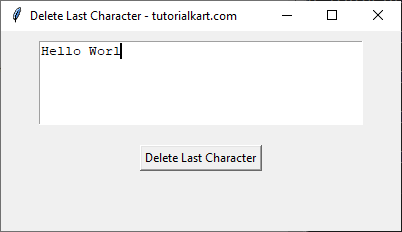
Conclusion
In this tutorial, we explored how to delete the last character in a Tkinter Text
widget using a button click to remove the last character.