How to Disable Entry Widget on Button Click in Tkinter Python
In Tkinter, an Entry
widget is used to take user input. You can disable the Entry
widget dynamically by setting its state to 'disabled'
. This can be done using the state
parameter or by calling the config()
method.
In this tutorial, we will explore multiple examples on how to disable an Entry
widget when a button is clicked in a Tkinter application.
Examples
1. Disabling Entry Widget on Button Click
In this example, we will create a simple Tkinter window where clicking a button will disable an entry field.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Disable Entry Example - tutorialkart.com")
root.geometry("400x200")
# Function to disable the entry field
def disable_entry():
entry.config(state="disabled")
# Create an entry widget
entry = tk.Entry(root)
entry.pack(pady=10)
# Create a button to disable the entry widget
btn_disable = tk.Button(root, text="Disable Entry", command=disable_entry)
btn_disable.pack(pady=10)
# Run the main event loop
root.mainloop()
Output in Windows:
A window appears as shown in the following.
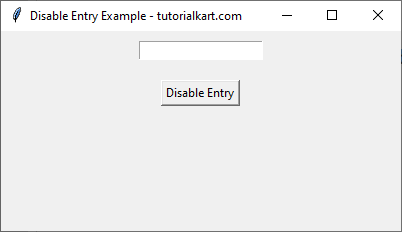
Enter some value in the Entry widget.
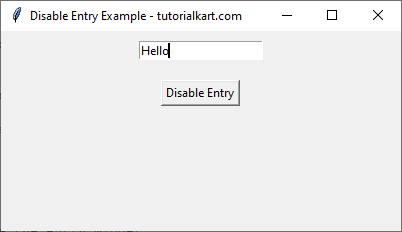
Click on Disable Entry button.
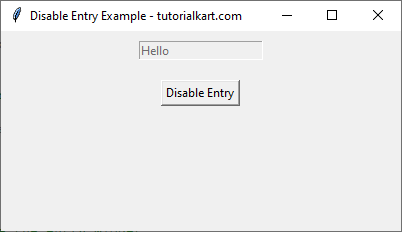
2. Toggling Between Enable and Disable
In this example, clicking the button will toggle between enabling and disabling the entry widget.
import tkinter as tk
root = tk.Tk()
root.title("Toggle Entry Example - tutorialkart.com")
root.geometry("400x200")
# Function to toggle entry state
def toggle_entry():
if entry["state"] == "normal":
entry.config(state="disabled")
btn_toggle.config(text="Enable Entry")
else:
entry.config(state="normal")
btn_toggle.config(text="Disable Entry")
# Create an entry widget
entry = tk.Entry(root)
entry.pack(pady=10)
# Create a button to toggle the entry widget
btn_toggle = tk.Button(root, text="Disable Entry", command=toggle_entry)
btn_toggle.pack(pady=10)
root.mainloop()
Output in Windows:
A window appears as shown in the following.
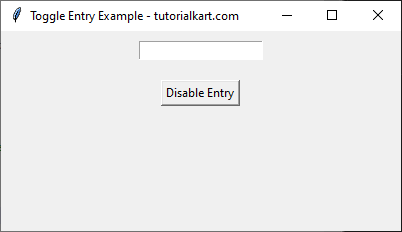
Enter a value in the Entry widget.
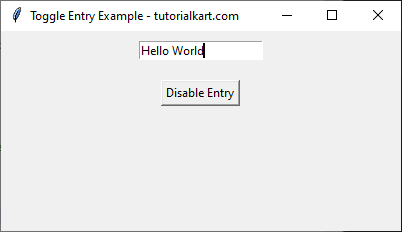
Click on Disable Entry button.
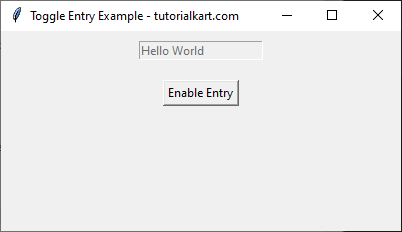
The Entry widget is disabled. You cannot change the value in the field in this state.
Click on Enable Entry button.
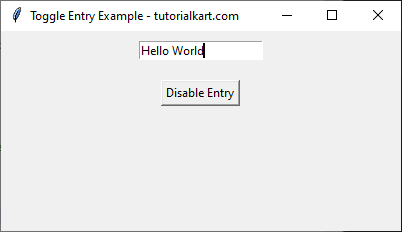
The Entry widget is enabled, and now you can change the value in it.
3. Disabling Entry After Submitting Text
In this example, the entry field will be disabled after the user enters text and clicks the “Submit” button.
import tkinter as tk
root = tk.Tk()
root.title("Submit & Disable Entry - tutorialkart.com")
root.geometry("400x200")
# Function to disable entry after submission
def submit_and_disable():
print("Submitted Text:", entry.get())
entry.config(state="disabled")
# Create an entry widget
entry = tk.Entry(root)
entry.pack(pady=10)
# Create a button to submit and disable entry
btn_submit = tk.Button(root, text="Submit", command=submit_and_disable)
btn_submit.pack(pady=10)
root.mainloop()
Output in Windows:
A window appears as shown in the following.
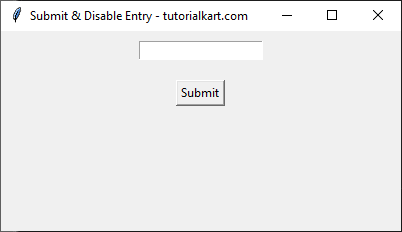
Enter a value, and click on the Submit button.
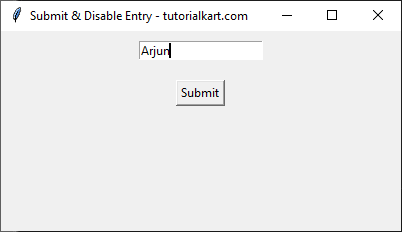
The value is read and printed to console output. Also, the Entry widget is disabled.
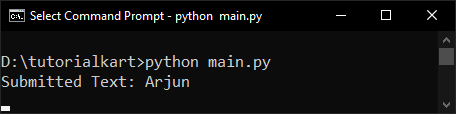
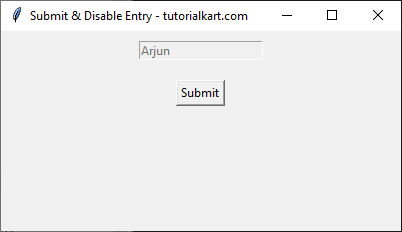
Conclusion
In this tutorial, we explored different ways to disable an Entry
widget using Tkinter:
- Using a button to disable the entry field.
- Toggling between enabling and disabling the entry field.
- Disabling the entry field after submitting text.