Disable Text Widget on Button Click in Tkinter Python
In Tkinter, the Text
widget is used for multi-line text input. To disable the Text
widget when a button is clicked, we can use the state
property and set it to DISABLED
. This prevents users from editing the text content.
The state
property of the Text
widget can have the following values:
NORMAL
– Allows editing (default state).DISABLED
– Prevents user input and disables the widget.
In this tutorial, we will explore different ways to disable the Text
widget using a Button in Tkinter.
Examples
1. Disable Text Widget on Button Click
In this example, we will create a Tkinter window with a Text
widget and a Button
. Clicking the button will disable the text widget, preventing further user input.
We create a disable_text()
function that sets the state of the Text
widget to DISABLED
. When the button is clicked, the function is executed using the command
parameter of the button.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Disable Text Widget - tutorialkart.com")
root.geometry("400x200")
# Function to disable text widget
def disable_text():
text_widget.config(state=tk.DISABLED)
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Create a Button to disable the Text widget
disable_button = tk.Button(root, text="Disable Text", command=disable_text)
disable_button.pack(pady=5)
# Run the main event loop
root.mainloop()
Output in Windows:
A text widget appears in the window.
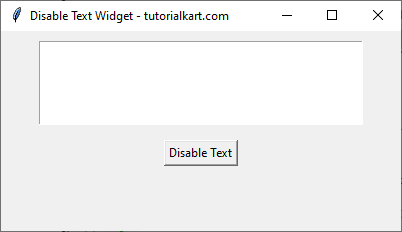
Let us enter some text into it.
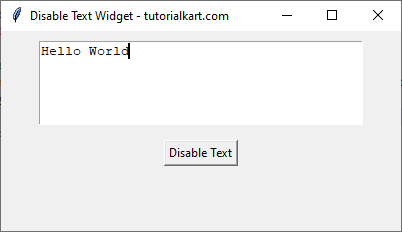
Let us click the Disable Text button.
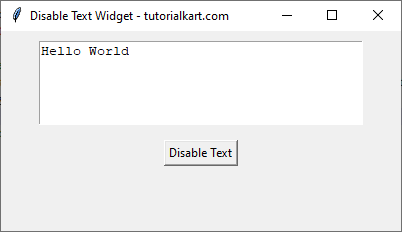
When the button is clicked, the text widget is disabled, preventing further input.
2. Toggle Between Enabling and Disabling the Text Widget
In this example, we will allow the user to toggle the text widget between enabled and disabled states using the same button.
We create a function toggle_text()
that checks the current state of the text widget. If it is NORMAL
, it is set to DISABLED
; otherwise, it is enabled back to NORMAL
. The button toggles between these two states.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Toggle Text Widget - tutorialkart.com")
root.geometry("400x200")
# Function to toggle the text widget state
def toggle_text():
if text_widget['state'] == tk.NORMAL:
text_widget.config(state=tk.DISABLED)
toggle_button.config(text="Enable Text")
else:
text_widget.config(state=tk.NORMAL)
toggle_button.config(text="Disable Text")
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Create a Button to toggle the Text widget state
toggle_button = tk.Button(root, text="Disable Text", command=toggle_text)
toggle_button.pack(pady=5)
# Run the main event loop
root.mainloop()
Output in Windows:
A text widget appears in the window along with a button labeled “Disable Text”.
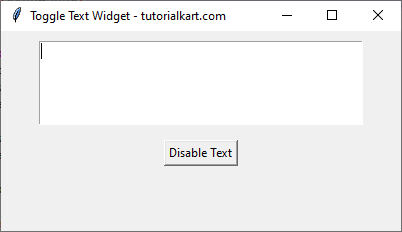
Enter some text into it.
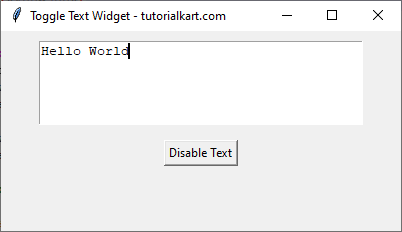
Click on Disable Text button. Clicking the button disables the text widget and changes the button label to “Enable Text”.
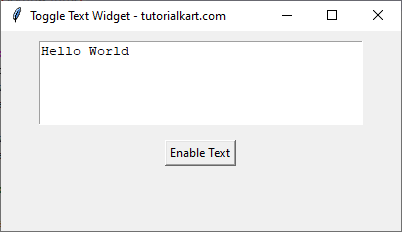
Now, clicking the Enable Text button enables the text widget.
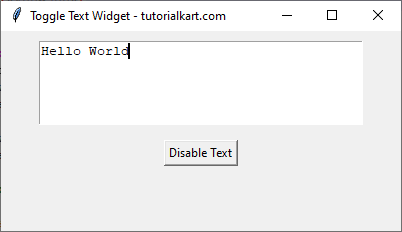
Conclusion
In this tutorial, we explored how to disable the Text
widget on button click using Tkinter. We covered:
- Disabling the
Text
widget using thestate
property. - Using a button to toggle between enabling and disabling the
Text
widget.