Disable Text Widget with a Default Value in Tkinter Python
In Tkinter, the Text
widget allows users to enter multi-line text. However, sometimes we need to disable the Text
widget to make it read-only while displaying a default value. This can be achieved using the state
option with the value DISABLED
.
To set a default value and disable the Text
widget:
- Insert the default text using
insert(INSERT, "Your text here")
- Disable editing by setting
state=tk.DISABLED
In this tutorial, we will explore different ways to set a default value in a Text
widget while keeping it disabled in a Tkinter application.
Examples
1. Creating a Disabled Text Widget with a Default Value
In this example, we create a Tkinter window with a Text
widget containing a default value, which is disabled to prevent user modification.
The program creates a Text
widget, inserts a default message using insert
, and then disables it using state=tk.DISABLED
. This ensures that users can read but not edit the text.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Text Widget Example - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Insert default text
text_widget.insert(tk.INSERT, "This is a read-only text field.")
# Disable the Text widget
text_widget.config(state=tk.DISABLED)
# Run the Tkinter event loop
root.mainloop()
Output in Windows:
A Text
widget appears with the default text “This is a read-only text field.”. The user cannot modify the text since the widget is disabled.
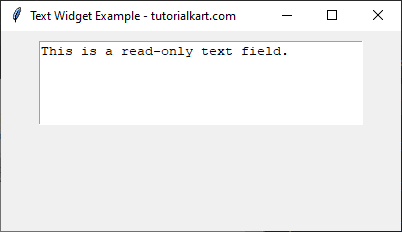
2. Enabling and Disabling Text Widget Dynamically
In this example, we create a Text
widget with a default value that starts as disabled but can be enabled using a button.
The program defines a function toggle_state()
that switches the Text
widget between enabled and disabled states. When enabled, the user can edit the text; when disabled, it becomes read-only again.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Toggle Text Widget - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Insert default text
text_widget.insert(tk.INSERT, "Click the button to enable editing.")
# Disable the Text widget
text_widget.config(state=tk.DISABLED)
# Function to toggle Text widget state
def toggle_state():
if text_widget.cget("state") == tk.DISABLED:
text_widget.config(state=tk.NORMAL)
else:
text_widget.config(state=tk.DISABLED)
# Create a button to toggle state
btn_toggle = tk.Button(root, text="Enable / Disable", command=toggle_state)
btn_toggle.pack(pady=10)
# Run the Tkinter event loop
root.mainloop()
Output in Windows:
A Text
widget appears with the default text “Click the button to enable editing.”. Initially, the widget is disabled.
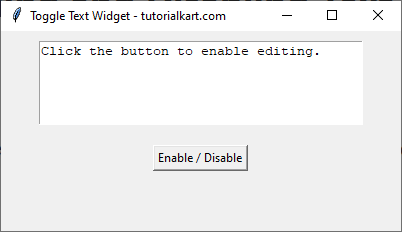
Clicking the button enables text editing, and clicking it again disables the widget.
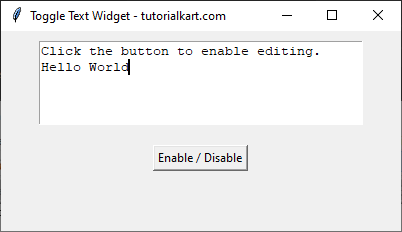
Conclusion
In this tutorial, we explored how to disable a Text
widget in Tkinter while displaying a default value. We also created an interactive example where the widget can be enabled and disabled dynamically. Using state=tk.DISABLED
, we ensure that users can view but not modify the text.