How to Disable the Button After Click in Tkinter
In Tkinter, you can disable a Button widget after it is clicked using the state
option. The state
attribute can be set to:
tk.NORMAL
– Enables the button (default state).tk.DISABLED
– Disables the button, preventing user interaction.
By setting the button state to tk.DISABLED
within the command function, you can disable it after the first click.
Examples
1. Disabling Button After Click
In this example, the button will be disabled once it is clicked.
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Disable Button Example - tutorialkart.com")
root.geometry("400x200")
def disable_button():
button.config(state=tk.DISABLED) # Disable the button after click
# Create a button
button = tk.Button(root, text="Click to Disable", command=disable_button)
button.pack(pady=20)
root.mainloop()
Output:
A button appears.
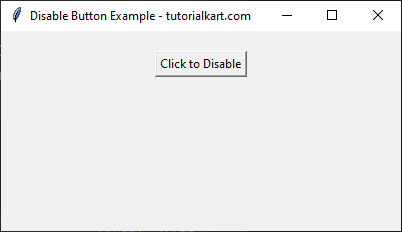
When clicked, it gets disabled and cannot be clicked again.
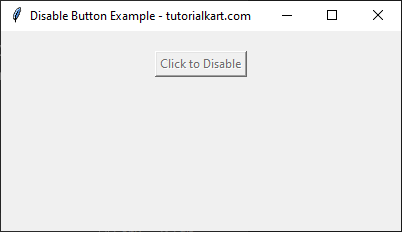
You can see that the text in the button has greyed out.
2. Disabling Button for a Few Seconds
In this example, the button will be disabled for 3 seconds after clicking and then re-enabled automatically.
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Temporary Disable Button - tutorialkart.com")
root.geometry("400x200")
def disable_temporarily():
button.config(state=tk.DISABLED) # Disable the button
root.after(3000, lambda: button.config(state=tk.NORMAL)) # Re-enable after 3 seconds
# Create a button
button = tk.Button(root, text="Click to Disable (3s)", command=disable_temporarily)
button.pack(pady=20)
root.mainloop()
Output:
A button appears.
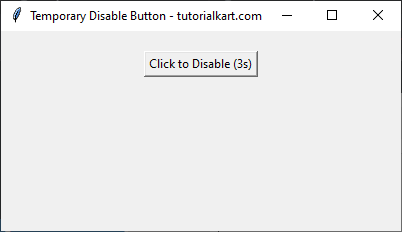
When clicked, it gets disabled for 3 seconds and then re-enables itself automatically.
Conclusion
In this tutorial, we learned how to disable a Button in Tkinter after clicking. You can:
- Permanently disable the button using
state=tk.DISABLED
. - Temporarily disable the button and re-enable it after a delay using
root.after()
.
These techniques help in preventing multiple button clicks in Tkinter applications.