Display Text Over an Image in Tkinter Python
In Tkinter, the Label
widget can be used to display both images and text together. This is achieved using the compound
option, which defines how the text and image are positioned relative to each other.
The compound
option can take the following values:
top
: Text is placed above the image.bottom
: Text is placed below the image.left
: Text is placed to the left of the image.right
: Text is placed to the right of the image.center
: Text is displayed on top of the image.
In this tutorial, we will learn how to use compound="center"
to display text over an image using the Label
widget in Tkinter.
Examples
1. Displaying Text Over the Image
In this example, we will display text directly on top of an image using the compound='center'
option.
</>
Copy
import tkinter as tk
from tkinter import PhotoImage
root = tk.Tk()
root.title("tutorialkart.com")
root.geometry("400x200")
# Load an image
image = PhotoImage(file="background-image.png") # Replace with an actual image path
# Create a Label with text over the image
label = tk.Label(root, text="Text Overlay", image=image, compound='center', fg="white", font=("Arial", 14, "bold"))
label.pack()
root.mainloop()
Output in Windows:
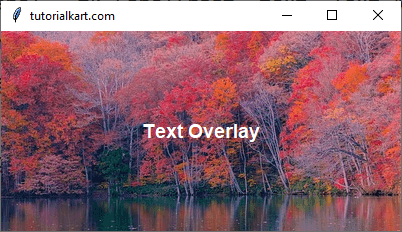
Conclusion
In this tutorial, we explored how to use the Label
widget in Tkinter to display text on images.