Focus Entry Widget in Tkinter Python
In Tkinter, the focus()
method is used to set the keyboard focus on an Entry
widget. When an Entry
widget is focused, the user can start typing into it without needing to click on it first.
To automatically focus an Entry
widget when the Tkinter window loads, we use the focus()
method inside the program. This ensures that the text input field is ready for user input as soon as the application opens.
In this tutorial, we will go through multiple examples demonstrating how to use the focus()
method in a Tkinter Entry widget.
Examples
1. Focusing on an Entry Widget at Startup
In this example, we create a simple Tkinter window with an Entry
widget that is focused as soon as the application starts.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Entry Focus Example - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget
entry = tk.Entry(root, font=("Arial", 14))
entry.pack(pady=20)
# Set focus on the Entry widget
entry.focus()
# Run the Tkinter main loop
root.mainloop()
Output in Windows:
The Entry widget is focused automatically when the window opens, allowing immediate typing.
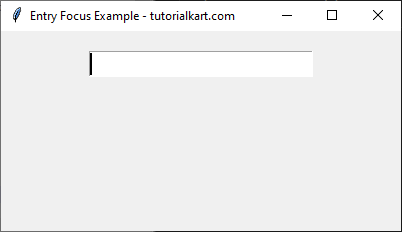
2. Focusing an Entry Widget When a Button is Clicked
In this example, the focus shifts to the Entry
widget when the button is clicked.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Button Click Focus Example - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget
entry = tk.Entry(root, font=("Arial", 14))
entry.pack(pady=10)
# Function to set focus on Entry widget
def focus_entry():
entry.focus()
# Create a button to trigger focus
btn_focus = tk.Button(root, text="Focus Entry", command=focus_entry)
btn_focus.pack(pady=10)
# Run the Tkinter main loop
root.mainloop()
Output in Windows:
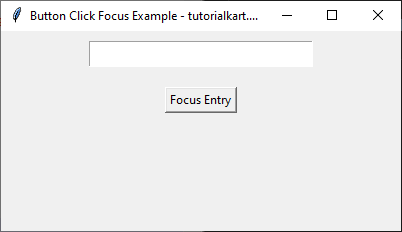
Clicking the “Focus Entry” button moves the cursor inside the Entry
widget, making it ready for input.
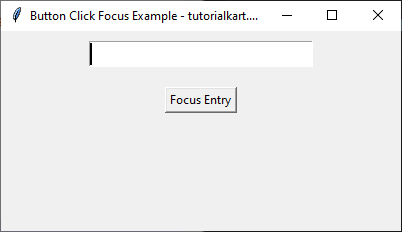
3. Auto-Focus on an Entry Widget After a Delay
In this example, the focus automatically moves to the Entry
widget after a delay of 3 seconds.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Delayed Focus Example - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget
entry = tk.Entry(root, font=("Arial", 14))
entry.pack(pady=20)
# Function to set focus on Entry widget after 3 seconds
root.after(3000, lambda: entry.focus())
# Run the Tkinter main loop
root.mainloop()
Output in Windows:
The Entry widget is focused automatically after 3 seconds when the window opens.
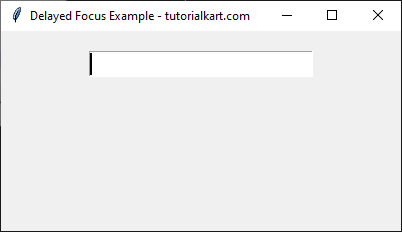
4. Switching Focus Between Multiple Entry Widgets
In this example, we create two Entry
widgets, and pressing the “Tab” button moves the focus between them.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Multiple Entry Focus Example - tutorialkart.com")
root.geometry("400x200")
# Create Entry widgets
entry1 = tk.Entry(root, font=("Arial", 14))
entry1.pack(pady=10)
entry2 = tk.Entry(root, font=("Arial", 14))
entry2.pack(pady=10)
# Set initial focus on the first Entry widget
entry1.focus()
# Run the Tkinter main loop
root.mainloop()
Output in Windows:
The first Entry widget is focused by default.
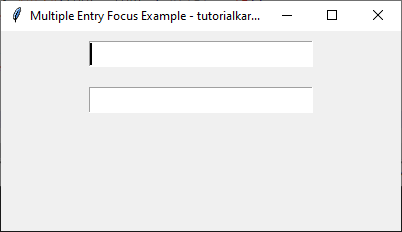
And pressing “Tab” moves focus to the second Entry widget.
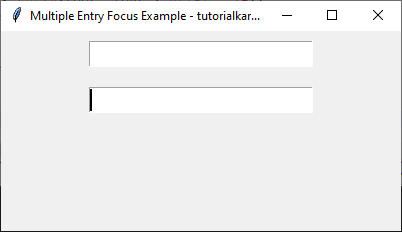
And pressing Tab again moves to the first Entry widget and so on.
Conclusion
In this tutorial, we explored different ways to use the focus()
method in Tkinter to set focus on an Entry widget. We covered:
- Automatically focusing an Entry widget at startup
- Setting focus when a button is clicked
- Delaying focus after a specific time
- Switching focus between multiple Entry widgets