Focus Text Widget in Tkinter Python
In Tkinter, the focus()
method is used to set focus on a widget. When a widget has focus, it can receive keyboard input. The Text
widget in Tkinter supports focusing, allowing users to start typing immediately without clicking on it.
To focus a Text
widget when the Tkinter window opens, you can call the focus()
method inside the main application script.
In this tutorial, we will go through examples of how to focus a Text widget in a Tkinter application.
Examples
1. Setting Focus on a Text Widget
In this example, we will create a Tkinter window with a Text
widget and set focus to it when the application starts.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Tkinter Focus Text Widget - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=20)
# Set focus to the Text widget
text_widget.focus()
# Run the main event loop
root.mainloop()
Output in Windows:
The Text widget receives focus automatically, allowing the user to start typing immediately without clicking on it.
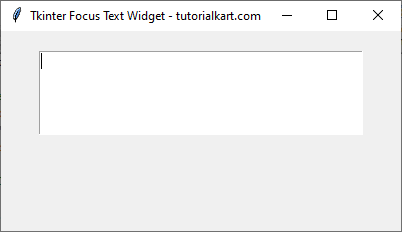
2. Setting Focus on a Text Widget Using a Button
In this example, we will create a Tkinter window with a Text
widget and a button. Clicking the button will set focus to the Text widget.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Tkinter Focus Text Widget - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Function to set focus to the Text widget
def focus_text():
text_widget.focus()
# Create a Button to set focus on the Text widget
btn_focus = tk.Button(root, text="Focus Text", command=focus_text)
btn_focus.pack(pady=10)
# Run the main event loop
root.mainloop()
Output in Windows:
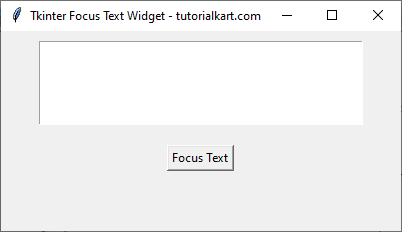
When the user clicks the “Focus Text” button, the Text widget gets focus, allowing them to start typing immediately.
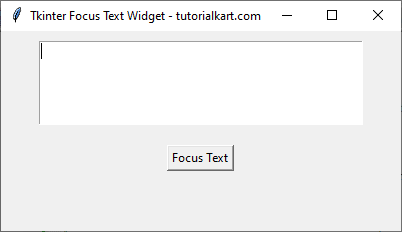
Conclusion
In this tutorial, we explored how to use the focus()
method in Tkinter to set focus on a Text widget. We covered:
- Automatically setting focus on the Text widget when the application starts.
- Using a button to programmatically set focus on the Text widget.