Get Cursor Position in Entry Widget in Tkinter Python
In Tkinter, the Entry
widget allows users to input text, and we can retrieve the cursor position using the index(tk.INSERT)
method. This method returns the current cursor position (caret) as an integer, indicating the index of the character where the cursor is placed.
The cursor position is useful when implementing text-processing features such as inserting text at the cursor, navigating text fields, or validating input dynamically.
Examples
1. Get Cursor Position on Button Click
In this example, we will create an Entry
widget and a button. When the button is clicked, the current cursor position inside the Entry
widget is displayed.
main.py
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Get Cursor Position - tutorialkart.com")
root.geometry("400x200")
# Function to get cursor position
def get_cursor_position():
position = entry.index(tk.INSERT)
label_result.config(text=f"Cursor Position: {position}")
# Create an Entry widget
entry = tk.Entry(root, font=("Arial", 14))
entry.pack(pady=20)
# Create a Button to get cursor position
btn_get_position = tk.Button(root, text="Get Cursor Position", command=get_cursor_position)
btn_get_position.pack(pady=10)
# Label to display cursor position
label_result = tk.Label(root, text="Cursor Position: ", font=("Arial", 12))
label_result.pack(pady=5)
root.mainloop()
Output in Windows:
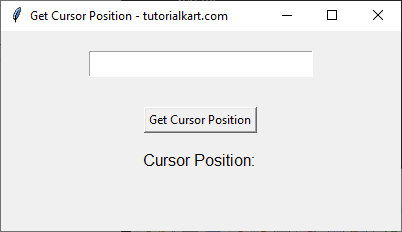
When the button is clicked, the cursor position in the Entry
widget is displayed.
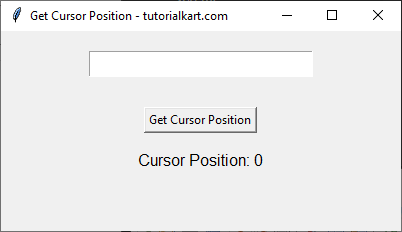
Since, the cursor is at initial position, the cursor position is 0. Let us enter some text, and then click on the button.
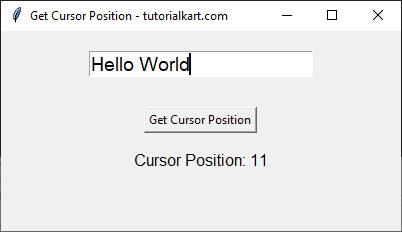
You may change the position of cursor, and then click the button, to get the current position of cursor.
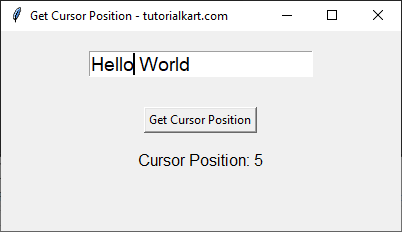
The cursor is right after the Hello word, which is 5.
2. Display Cursor Position in Real-Time
In this example, we will continuously update and display the cursor position in real-time as the user moves the cursor inside the Entry
widget.
main.py
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Real-Time Cursor Position - tutorialkart.com")
root.geometry("400x200")
# Function to update cursor position in real-time
def update_cursor_position(event):
position = entry.index(tk.INSERT)
label_result.config(text=f"Cursor Position: {position}")
# Create an Entry widget
entry = tk.Entry(root, font=("Arial", 14))
entry.pack(pady=20)
entry.bind("<KeyRelease>", update_cursor_position)
entry.bind("<ButtonRelease>", update_cursor_position)
# Label to display cursor position
label_result = tk.Label(root, text="Cursor Position: 0", font=("Arial", 12))
label_result.pack(pady=10)
root.mainloop()
Output in Windows:
The cursor position updates dynamically as the user moves the cursor inside the Entry
widget.
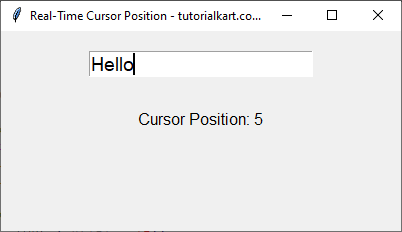
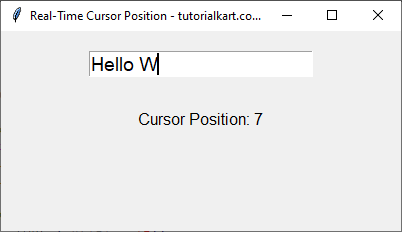
Conclusion
In this tutorial, we explored how to get the cursor position inside a Tkinter Entry
widget. We used the index(tk.INSERT)
method to retrieve the cursor’s location and implemented two different approaches:
- Getting the cursor position when a button is clicked.
- Displaying the cursor position in real-time as the user types or moves the cursor.