Cursor Position in Text Widget
In Tkinter, the Text
widget allows users to enter multiple lines of text. To get the cursor position within a Text widget, you can use the index
method, which returns the row and column position of the insertion cursor.
By retrieving the cursor position dynamically, we can implement interactive features such as displaying the cursor location, triggering actions based on position, or performing text operations.
Examples
1. Display Cursor Position in Tkinter Text Widget
In this example, we create a Tkinter window with a Text
widget and a Label
. The cursor position is updated dynamically as the user moves within the text widget.
We define a function update_position
that retrieves the cursor position using text_widget.index("insert")
and updates the label with the current row and column. The Text
widget uses the <KeyRelease>
event to trigger this function whenever a key is pressed.
main.py
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Cursor Position in Text Widget - tutorialkart.com")
root.geometry("400x200")
def update_position(event):
"""Update label with the cursor's current position."""
position = text_widget.index("insert") # Get cursor position
lbl_position.config(text=f"Cursor Position: {position}")
# Create Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Create Label to display cursor position
lbl_position = tk.Label(root, text="Cursor Position: 1.0")
lbl_position.pack(pady=5)
# Bind KeyRelease event to track cursor movement
text_widget.bind("<KeyRelease>", update_position)
# Run application
root.mainloop()
Output in Windows:
A text area appears where users can type.
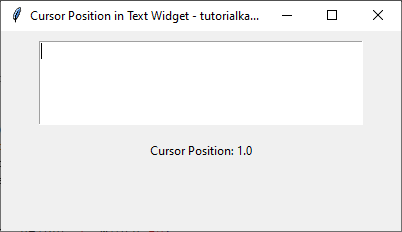
Current Position: 1.0. Where 1 is for row, and 0 is for column.
As the user moves the cursor or types, the label below dynamically updates, showing the current cursor position.
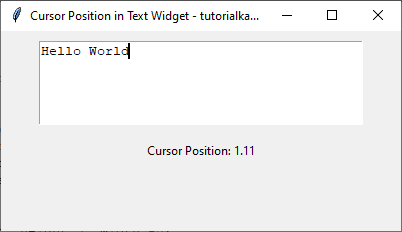
Current Position: 1.0. Where 1 is for row, and 11 is for column.
Using mouse, you can click at another position, and the cursor position should be updated.
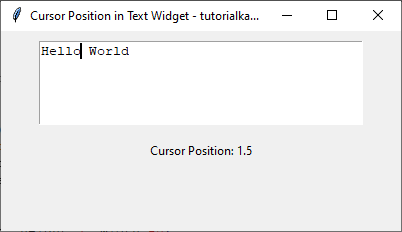
Now, cursor’s Current Position: 1.5. Where 1 is for row, and 5 is for column.
2. Get Cursor Position in Text Widget on Button Click
In this example, we create a Text
widget and a Button
. When the button is clicked, it fetches and displays the cursor position in a label.
We use the function get_cursor_position
which retrieves the cursor’s location with text_widget.index("insert")
. The button is set to call this function when clicked, and the label updates with the cursor’s current position.
main.py
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Get Cursor Position on Button Click - tutorialkart.com")
root.geometry("400x200")
def get_cursor_position():
"""Fetch and display cursor position when button is clicked."""
position = text_widget.index("insert") # Get cursor position
lbl_position.config(text=f"Cursor Position: {position}")
# Create Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Create Button to fetch cursor position
btn_get_position = tk.Button(root, text="Get Cursor Position", command=get_cursor_position)
btn_get_position.pack(pady=5)
# Create Label to display cursor position
lbl_position = tk.Label(root, text="Cursor Position: ")
lbl_position.pack(pady=5)
# Run application
root.mainloop()
Output in Windows:
A text box and a button appear. When the user clicks the button, the label updates with the cursor’s current position in the text widget.
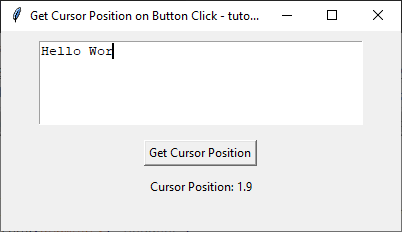
Conclusion
In this tutorial, we explored different ways to get the cursor position in a Tkinter Text
widget. The index("insert")
method is essential for retrieving the row and column of the cursor. We used event binding to update the position dynamically and a button click to fetch the position on demand.