Get Text Entered in Entry Widget in Tkinter Python
In Tkinter, the Entry
widget is used to accept single-line text input from the user. To retrieve the text entered in an Entry widget, we use the get()
method.
The get()
method returns the current text entered by the user in the Entry field as a string. It is commonly used in event-driven applications where user input is required.
In this tutorial, we will explore multiple examples of using the get()
method to retrieve user input from an Entry widget in a Tkinter application.
Examples
1. Retrieving Text from Entry Widget
In this example, we create a Tkinter window with an Entry widget and a button. When the button is clicked, the entered text is displayed in the console.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Get Entry Text Example - tutorialkart.com")
root.geometry("400x200")
def get_text():
user_input = entry.get() # Retrieve text from Entry widget
print("Entered Text:", user_input)
# Create an Entry widget
entry = tk.Entry(root, width=30)
entry.pack(pady=10)
# Create a Button to retrieve text
btn = tk.Button(root, text="Get Text", command=get_text)
btn.pack(pady=5)
root.mainloop()
Output in Windows:
A window appears with an Entry field and a button labeled “Get Text”.
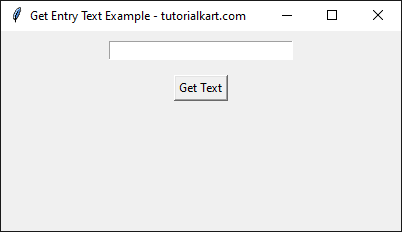
When you enter text and click the button, the entered text is printed in the console.
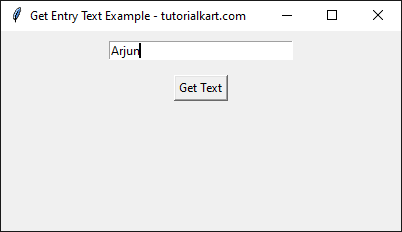
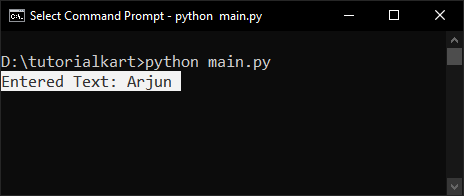
2. Displaying Entered Text in a Label
Instead of printing the text in the console, we will display it inside a Label widget.
import tkinter as tk
root = tk.Tk()
root.title("Display Entry Text - tutorialkart.com")
root.geometry("400x200")
def display_text():
user_input = entry.get()
label.config(text="Entered: " + user_input)
entry = tk.Entry(root, width=30)
entry.pack(pady=10)
btn = tk.Button(root, text="Show Text", command=display_text)
btn.pack(pady=5)
label = tk.Label(root, text="Entered: ")
label.pack(pady=10)
root.mainloop()
Output in Windows:
A window appears with an Entry field, a button labeled “Show Text,” and a label.
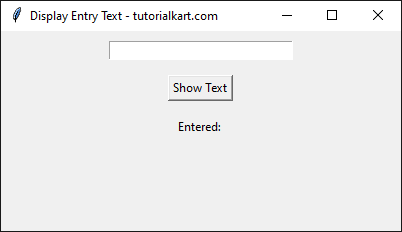
When text is entered and the button is clicked, the entered text is displayed in the Label.
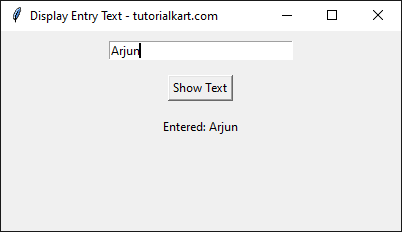
3. Clearing the Entry Field After Retrieving Text
In this example, we retrieve the entered text and clear the Entry field after the button is clicked.
import tkinter as tk
root = tk.Tk()
root.title("Clear Entry After Get - tutorialkart.com")
root.geometry("400x200")
def get_and_clear():
user_input = entry.get()
print("Entered Text:", user_input)
entry.delete(0, tk.END) # Clear Entry field
entry = tk.Entry(root, width=30)
entry.pack(pady=10)
btn = tk.Button(root, text="Get & Clear", command=get_and_clear)
btn.pack(pady=5)
root.mainloop()
Output in Windows:
A window appears with an Entry field and a button labeled “Get & Clear”.
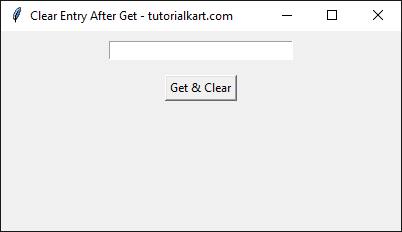
When text is entered and the button is clicked, the text is printed in the console, and the Entry field is cleared.
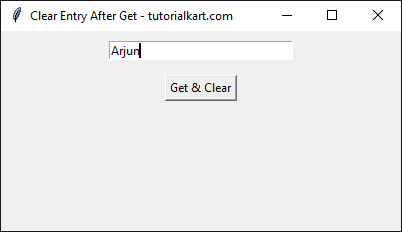
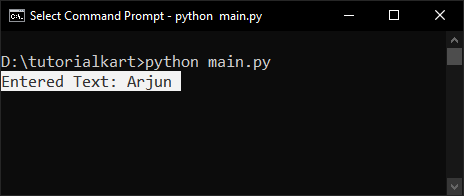
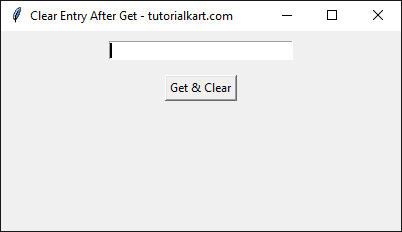
4. Using a Text Variable to Retrieve Input from Entry Field
Instead of using the get()
method, we can bind an Entry widget to a StringVar variable to retrieve text dynamically.
import tkinter as tk
root = tk.Tk()
root.title("Entry with Variable - tutorialkart.com")
root.geometry("400x200")
text_var = tk.StringVar()
def show_text():
label.config(text="Entered: " + text_var.get())
entry = tk.Entry(root, textvariable=text_var, width=30)
entry.pack(pady=10)
btn = tk.Button(root, text="Show Text", command=show_text)
btn.pack(pady=5)
label = tk.Label(root, text="Entered: ")
label.pack(pady=10)
root.mainloop()
Output in Windows:
A window appears with an Entry field and a button labeled “Show Text.” When text is entered, clicking the button dynamically updates the label with the entered text.
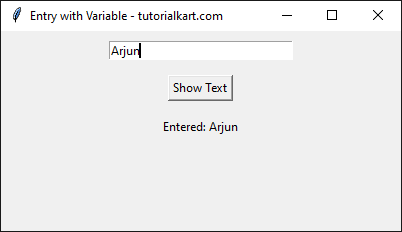
Conclusion
In this tutorial, we explored different ways to retrieve text entered in an Entry widget using Tkinter. The get()
method and StringVar
provide different ways to manage user input:
- Using
get()
to retrieve text when a button is clicked. - Displaying the entered text in a Label widget.
- Clearing the Entry field after retrieving text.
- Using a
StringVar
variable for real-time text updates.