Get Value Entered in Text Widget in Tkinter Python
In Tkinter, the Text
widget is used to accept multi-line user input. To retrieve the value entered in the Text
widget, the get()
method is used with a specific range of indices.
The syntax to retrieve text is:
text_widget.get("start_index", "end_index")
where:
start_index
is the starting position (e.g.,"1.0"
for the first character of the first line).end_index
is the ending position (e.g.,"end"
to retrieve all text).
In this tutorial, we will explore different ways to retrieve the value entered in the Tkinter Text
widget with detailed examples.
Examples
1. Getting Text from Text Widget on Button Click
In this example, we will create a simple Tkinter application where the user enters text in a Text
widget, and when they click the button, the entered text is displayed in the console.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Text Widget Example - tutorialkart.com")
root.geometry("400x200")
# Function to get text from Text widget
def get_text():
entered_text = text_box.get("1.0", "end-1c") # Exclude the trailing newline
print("Entered Text:", entered_text)
# Create a Text widget
text_box = tk.Text(root, height=5, width=40)
text_box.pack(pady=10)
# Create a button to fetch text
btn_get = tk.Button(root, text="Get Text", command=get_text)
btn_get.pack(pady=5)
root.mainloop()
Output in Windows:
A window appears with a multi-line text box and a button labeled “Get Text”.
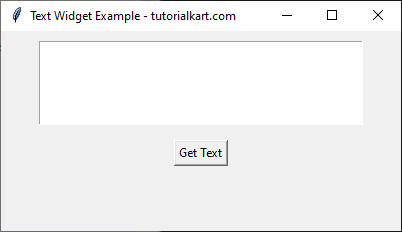
Enter some text into the Text box.
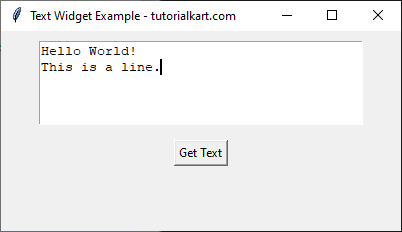
When the button is clicked, the text entered in the Text
widget is printed in the console.
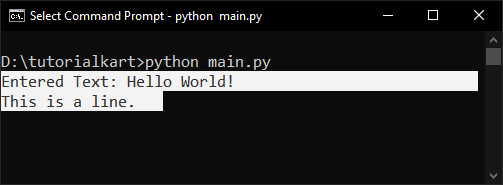
2. Displaying Entered Text in a Label
In this example, we will retrieve the text from the Text
widget and display it inside a Label
widget instead of printing it in the console.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Display Text Example - tutorialkart.com")
root.geometry("400x200")
# Function to get text and display in label
def display_text():
entered_text = text_box.get("1.0", "end-1c") # Remove trailing newline
label_output.config(text="You Entered: " + entered_text)
# Create a Text widget
text_box = tk.Text(root, height=5, width=40)
text_box.pack(pady=10)
# Create a button to fetch text
btn_display = tk.Button(root, text="Show Text", command=display_text)
btn_display.pack(pady=5)
# Create a Label to display entered text
label_output = tk.Label(root, text="", fg="blue")
label_output.pack(pady=5)
root.mainloop()
Output in Windows:
A window appears with a multi-line text box, a button labeled “Show Text”, and an empty label.
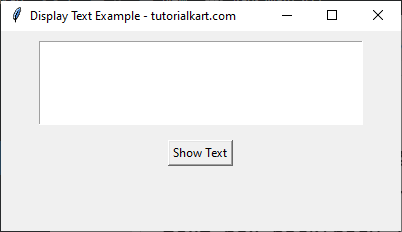
When the button is clicked, the entered text is displayed inside the label.
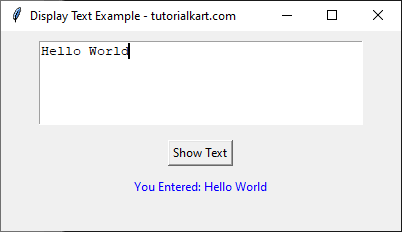
Conclusion
In this tutorial, we explored how to retrieve values entered in a Tkinter Text
widget using the get()
method. We covered two examples:
- Printing the entered text in the console.
- Displaying the entered text inside a
Label
widget.