Undo and Redo for Entry Widget in Tkinter Python
In Tkinter, the Entry
widget allows users to input text. However, the built-in undo=True
option is not supported in Python 3.12. To implement undo and redo functionality, we can create a custom mechanism using a stack-based approach.
In this tutorial, we will demonstrate how to implement undo and redo for an Entry
widget by tracking changes and storing them in separate stacks.
Example: Implementing Custom Undo and Redo in Tkinter Entry
In this example, we will create an Entry widget that supports undo and redo using buttons and keyboard shortcuts.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Undo and Redo Example - tutorialkart.com")
root.geometry("400x200")
# Stack to store text history
undo_stack = [""]
redo_stack = []
# Function to update undo stack on text change
def on_text_change(event=None):
current_text = entry_widget.get()
if undo_stack[-1] != current_text:
undo_stack.append(current_text)
# Clear redo stack on new input
redo_stack.clear()
# Function to handle undo
def undo_action(event=None):
if len(undo_stack) > 1:
redo_stack.append(undo_stack.pop())
entry_widget.delete(0, tk.END)
entry_widget.insert(0, undo_stack[-1])
# Function to handle redo
def redo_action(event=None):
if redo_stack:
text = redo_stack.pop()
undo_stack.append(text)
entry_widget.delete(0, tk.END)
entry_widget.insert(0, text)
# Create an Entry widget
entry_widget = tk.Entry(root, width=40, font=("Arial", 12))
entry_widget.pack(pady=20)
# Capture every key press to track changes
entry_widget.bind("<KeyRelease>", on_text_change)
# Create Undo and Redo buttons
btn_undo = tk.Button(root, text="Undo", command=undo_action)
btn_undo.pack(side="left", padx=20)
btn_redo = tk.Button(root, text="Redo", command=redo_action)
btn_redo.pack(side="right", padx=20)
# Bind keyboard shortcuts
root.bind("<Control-z>", undo_action)
root.bind("<Control-y>", redo_action)
# Run the Tkinter event loop
root.mainloop()
Output in Windows:
Running the application opens the following window.
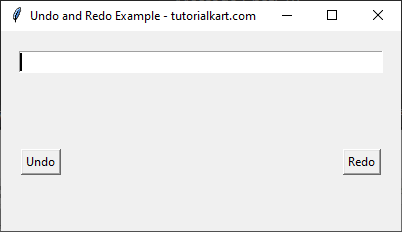
Start typing some text into the Entry field.
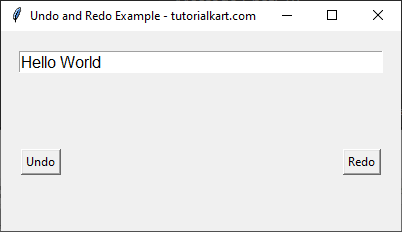
Now, click on the Undo button.
Click Undo (or press Ctrl+Z
) to revert to the last text state.
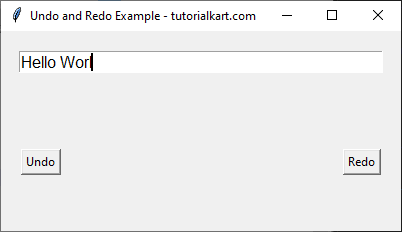
Click Redo (or press Ctrl+Y
) to restore undone changes.
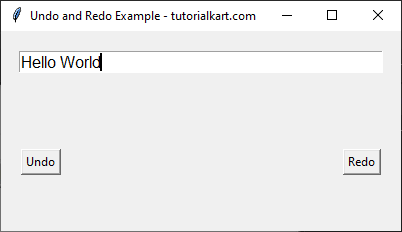
Explanation
- Undo Stack: Stores the previous states of the text.
- Redo Stack: Stores undone text for reapplying.
on_text_change()
: Tracks input changes and updates the undo stack.undo_action()
: Pops the last text from the undo stack, stores the current text in the redo stack, and restores the last state.redo_action()
: Pops the last undone text from the redo stack and restores it.- Keyboard Shortcuts:
Ctrl+Z
(Undo) andCtrl+Y
(Redo) for easier use.
Conclusion
Since the built-in undo=True
option is not available in Python 3.12, we implemented a custom undo and redo mechanism using stacks. This approach ensures that users can revert and reapply text changes easily using buttons or keyboard shortcuts.