Justify Text in Label in Tkinter Python
In Tkinter, the justify
option of a Label widget allows you to control the alignment of multi-line text within the label. The available justification values are:
LEFT
– Aligns text to the left.CENTER
– Centers text within the label.RIGHT
– Aligns text to the right.
By default, the text inside a Label is left-aligned. In this tutorial, we will go through multiple examples demonstrating how to justify text in a Tkinter Label.
Examples
1. Left Justified Text in Label
In this example, we will create a Label with left-justified text.
import tkinter as tk
root = tk.Tk()
root.title("Justify Text in Label - tutorialkart.com")
root.geometry("400x200")
# Create a left-justified label
label = tk.Label(root, text="This is an example of\nleft-justified text.", justify=tk.LEFT)
label.pack(pady=10)
root.mainloop()
Output in Windows:
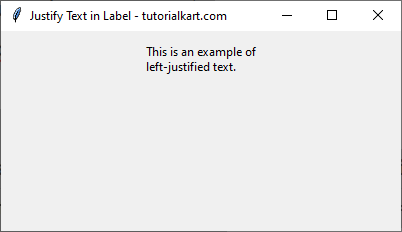
2. Center Justified Text in Label
In this example, we will create a Label with center-justified text.
import tkinter as tk
root = tk.Tk()
root.title("Justify Text in Label - tutorialkart.com")
root.geometry("400x200")
# Create a center-justified label
label = tk.Label(root, text="This is an example of\ncenter-justified text.", justify=tk.CENTER)
label.pack(pady=10)
root.mainloop()
Output in Windows:
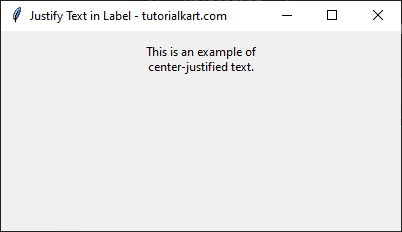
3. Right Justified Text in Label
In this example, we will create a Label with right-justified text.
import tkinter as tk
root = tk.Tk()
root.title("Justify Text in Label - tutorialkart.com")
root.geometry("400x200")
# Create a right-justified label
label = tk.Label(root, text="This is an example of\nright-justified text.", justify=tk.RIGHT)
label.pack(pady=10)
root.mainloop()
Output in Windows:
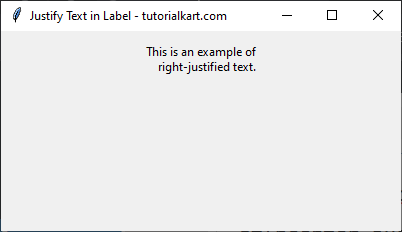
Conclusion
In this tutorial, we explored how to use the justify
option in Tkinter’s Label widget to align text. The text can be:
- Left-justified using
justify=tk.LEFT
- Center-justified using
justify=tk.CENTER
- Right-justified using
justify=tk.RIGHT
By setting different justification values, you can adjust the alignment of multi-line text within your Tkinter application.