Left Align Text in Label in Tkinter Python
In Tkinter, the Label
widget is used to display text or images. By default, the text inside a Label is centered, but we can left-align the text using the anchor
and justify
options.
The anchor
option is used to align the text within the Label’s bounding box, while the justify
option controls the alignment of multiple lines of text.
In this tutorial, we will go through examples demonstrating how to left-align text inside a Tkinter Label.
Examples
1. Left Aligning Single Line Text in Label
In this example, we use the anchor="w"
option to left-align a single line of text inside a Label.
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Left Align Label Example")
root.geometry("400x200")
# Create a label with left-aligned text
label = tk.Label(root, text="This text is left-aligned", anchor="w", width=40)
label.pack(pady=10, padx=20, fill="x")
root.mainloop()
Output in Windows:
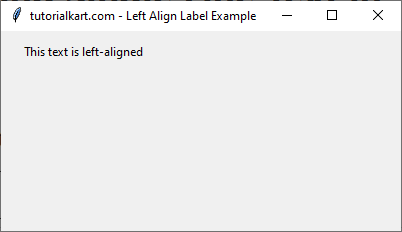
2. Left Aligning Multi-line Text in Label
For multi-line text, we use both anchor="w"
and justify="left"
to ensure that all lines are left-aligned.
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Left Align Multi-line Label")
root.geometry("400x200")
# Create a multi-line label with left-aligned text
label = tk.Label(root, text="This is a multi-line\nleft-aligned text\nin Tkinter Label.",
anchor="w", justify="left", width=40)
label.pack(pady=10, padx=20, fill="x")
root.mainloop()
Output in Windows:
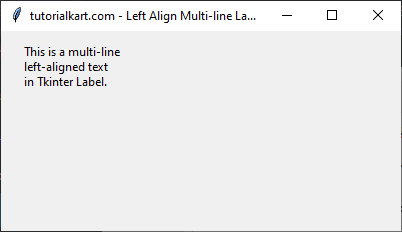
3. Left Aligning Label with a Fixed Width and Background Color
We can set a background color to visualize the left alignment more clearly.
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Label Alignment with Background")
root.geometry("400x200")
# Create a label with left-aligned text and background color
label = tk.Label(root, text="Left-aligned text with background",
anchor="w", width=40, bg="lightgray")
label.pack(pady=10, padx=20, fill="x")
root.mainloop()
Output in Windows:
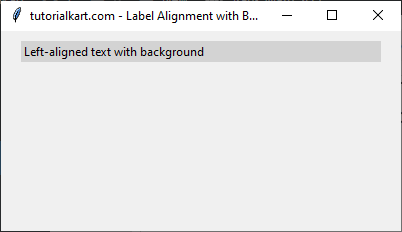
Conclusion
In this tutorial, we explored how to left-align text in a Tkinter Label using:
anchor="w"
for single-line text alignmentanchor="w"
andjustify="left"
for multi-line text alignment- Using a background color to enhance visibility
By using these techniques, you can better control the text alignment inside a Tkinter Label for improved readability.