Limit Characters in Entry Widget in Tkinter Python
In Tkinter, the Entry
widget is used to take user input. By default, the user can enter an unlimited number of characters. However, in many applications, you may need to limit the number of characters that can be entered into the Entry widget.
To limit characters in a Tkinter Entry widget, you can use the validate
and validatecommand
options along with a function that restricts input based on character length.
In this tutorial, we will go through various examples using different methods to limit characters in an Entry widget in Tkinter.
Examples
1. Limiting Characters in Entry Widget Using validate and validatecommand
In this example, we use the validate
option set to 'key'
and a function that ensures the input length does not exceed a defined limit.
import tkinter as tk
# Function to validate input length
def limit_input_length(input_text):
if len(input_text) > 10:
return False
return True
root = tk.Tk()
root.title("tutorialkart.com - Limit Entry Characters")
root.geometry("400x200")
# Register validation function
vcmd = root.register(limit_input_length)
# Create Entry widget with validation
entry = tk.Entry(root, validate="key", validatecommand=(vcmd, "%P"))
entry.pack(pady=20)
root.mainloop()
Output in Windows:
The Entry widget allows a maximum of 10 characters to be entered.
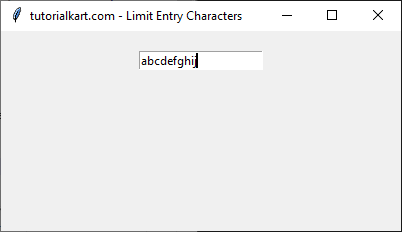
If you try to enter more than 10 characters, nothing happens in the Entry widget, it just remains as if you have input nothing.
2. Limiting Characters in Entry Widget Using trace
Method
Another way to restrict character entry is by using Tkinter’s trace
method, which monitors variable changes.
import tkinter as tk
def limit_entry(*args):
value = entry_text.get()
if len(value) > 8:
entry_text.set(value[:8]) # Trim input to 8 characters
root = tk.Tk()
root.title("tutorialkart.com - Limit Entry Characters")
root.geometry("400x200")
# StringVar to track input
entry_text = tk.StringVar()
entry_text.trace("w", limit_entry)
# Create Entry widget
entry = tk.Entry(root, textvariable=entry_text)
entry.pack(pady=20)
root.mainloop()
Output in Windows:
The input is automatically trimmed to a maximum of 8 characters.
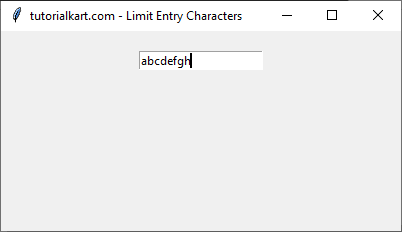
3. Blocking Input After Character Limit
This method prevents further input when the limit is reached.
import tkinter as tk
def check_length(event):
if len(entry.get()) >= 6:
entry.delete(5, tk.END) # Remove extra characters
root = tk.Tk()
root.title("tutorialkart.com - Limit Entry Characters")
root.geometry("400x200")
# Create Entry widget and bind keypress event
entry = tk.Entry(root)
entry.pack(pady=20)
entry.bind("<KeyRelease>", check_length)
root.mainloop()
Output in Windows:
The user can type a maximum of 6 characters. Any extra characters that are more than five are removed instantly.
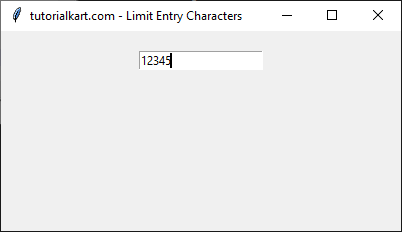
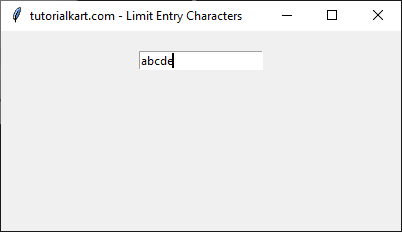
This allowing of six characters to type, and then keeping only the five characters gives user a kind of feedback that a maximum of only five characters are allowed.
Conclusion
In this tutorial, we explored different ways to limit the number of characters in an Entry widget in Tkinter:
- Using
validate
andvalidatecommand
to restrict input dynamically. - Using
trace
to monitor changes and adjust input automatically. - Using key event bindings to prevent excess characters.
By implementing these techniques, you can control user input effectively in your Tkinter applications.