Limit Characters in Text Widget in Tkinter Python
In Tkinter, the Text
widget allows users to input multi-line text. However, by default, there is no built-in feature to limit the number of characters a user can enter. To achieve this, we can use event binding and the INSERT
index to control the user input.
In this tutorial, we will explore different methods to limit the number of characters in a Tkinter Text Widget using event handling.
Examples
1. Restricting Text Entry to a Maximum Number of Characters
In this example, we will create a Tkinter Text
widget and limit the input to a maximum of 30 characters.
import tkinter as tk
def limit_text(event):
"""Prevent entering more than the allowed limit."""
max_chars = 50
text = text_widget.get("1.0", "end-1c") # Get text excluding last newline
if len(text) >= max_chars and event.keysym not in ("BackSpace", "Delete"):
return "break" # Prevent further input
# Create main window
root = tk.Tk()
root.title("Limit Characters in Text Widget - tutorialkart.com")
root.geometry("400x200")
# Create Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Bind event to limit character input
text_widget.bind("<KeyPress>", limit_text)
root.mainloop()
We have bind the key-press event to the text widget with the limit_text()
function. When ever the user presses a key to enter input in the text widget, the event is triggered and the limit_text()
function is called. Inside this limit_text()
function, we are validating the length of input text in the text widget, and truncating if the length is more than allowed limit on number of characters.
Output in Windows:
Initial screen.
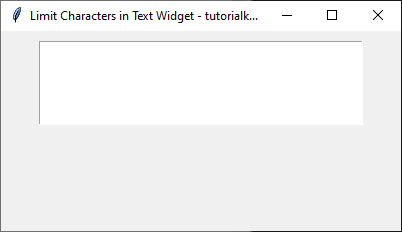
You may start typing some content in the text widget.
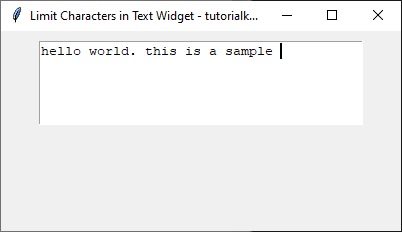
The Text widget prevents input beyond 30 characters while still allowing Backspace and Delete keys.
2. Showing Remaining Characters While Typing in a Text Widget
In this example, we will display a label showing the remaining characters allowed as the user types.
import tkinter as tk
def update_label(event):
"""Update remaining characters count and prevent exceeding limit."""
max_chars = 50
text = text_widget.get("1.0", "end-1c") # Get text excluding last newline
remaining = max_chars - len(text)
if remaining < 0 and event.keysym not in ("BackSpace", "Delete"):
return "break" # Prevent further input
char_label.config(text=f"Characters remaining: {max(0, remaining)}")
# Create main window
root = tk.Tk()
root.title("Limit Characters in Text Widget - tutorialkart.com")
root.geometry("400x200")
# Create Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Create label to show remaining characters
char_label = tk.Label(root, text="Characters remaining: 50")
char_label.pack()
# Bind event to update character count
text_widget.bind("<KeyPress>", update_label)
root.mainloop()
Output in Windows:
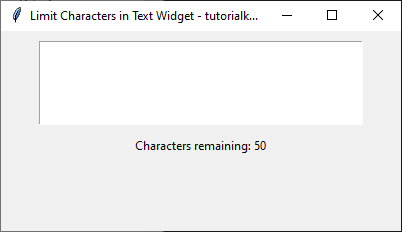
The Text widget displays the number of characters remaining, preventing input beyond 50 characters. This helps to give feedback to the user about the number of character remaining.
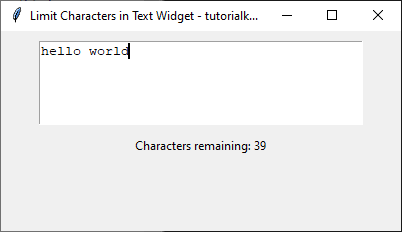
Conclusion
In this tutorial, we explored how to limit the number of characters in a Tkinter Text
widget. We covered:
- Restricting the number of characters a user can enter.
- Displaying the remaining character count dynamically.