Right Align Text in Entry Widget in Tkinter Python
In Tkinter, the justify
option of the Entry
widget allows you to align the text inside the input field. By default, text is left-aligned, but you can set it to RIGHT
to align it to the right.
To right-align text in an Entry widget, use the following syntax:
tk.Entry(root, justify="right")
In this tutorial, we will go through detailed examples of how to right-align text in the Entry widget in Tkinter.
Examples
1. Right Aligning Text in Entry Widget
In this example, we will create a simple Tkinter application where an Entry widget contains right-aligned text.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Right Align Entry - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget with right-aligned text
entry = tk.Entry(root, justify="right", font=("Arial", 14))
entry.insert(0, "Enter your name")
entry.pack(pady=20, padx=20, fill="x")
# Run the main event loop
root.mainloop()
Output in Windows:
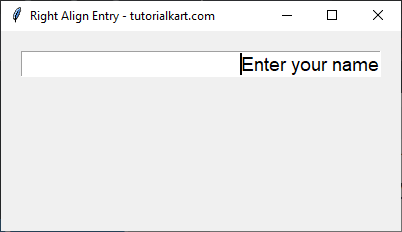
Remove the default text and start entering your name.
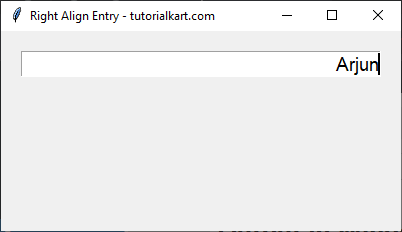
2. Right Aligning Entry Widget with Label
In this example, we will create a Tkinter application with an Entry widget and a corresponding Label. The text inside the Entry field will be right-aligned.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Right Align Entry - tutorialkart.com")
root.geometry("400x200")
# Create a Label
label = tk.Label(root, text="Amount:", font=("Arial", 12))
label.pack(pady=5, padx=20, anchor="w")
# Create an Entry widget with right-aligned text
entry = tk.Entry(root, justify="right", font=("Arial", 14))
entry.insert(0, "1000")
entry.pack(pady=5, padx=20, fill="x")
# Run the main event loop
root.mainloop()
Output in Windows:
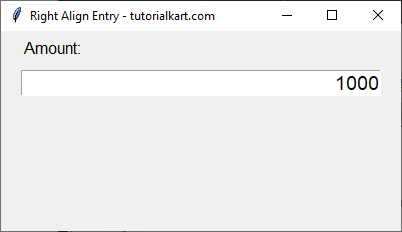
Conclusion
In this tutorial, we explored how to use the justify
option in Tkinter’s Entry widget to align text to the right. The justify
option can take values "left"
, "center"
, or "right"
. Right-aligning is useful for numerical inputs such as amounts, prices, or calculations.