Right Align Text in Label in Tkinter Python
In Tkinter, the anchor
and justify
options of the Label
widget allow you to align text inside the label. The anchor
option is used for positioning text within the label’s bounding box, while the justify
option controls text alignment in multi-line labels.
To right-align text in a Tkinter Label, set anchor='e'
for single-line text and justify='right'
for multi-line text.
In this tutorial, we will go through examples to demonstrate how to right-align text in a Tkinter Label.
Examples
1. Right Aligning Single-Line Text
In this example, we use the anchor='e'
option to right-align a single-line text inside a label.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Right Align Label - tutorialkart.com")
root.geometry("400x200")
# Create a right-aligned label
label = tk.Label(root, text="Right Aligned Text", anchor="e", width=40)
label.pack(pady=20)
root.mainloop()
Output in Windows:
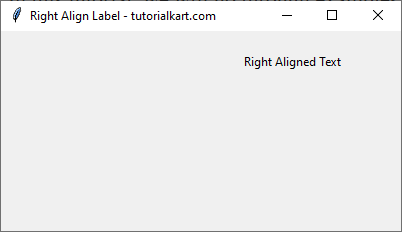
2. Right Aligning Multi-Line Text
In this example, we use the justify='right'
option to right-align a multi-line text inside a label.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Right Align Multi-Line Label - tutorialkart.com")
root.geometry("400x200")
# Create a right-aligned multi-line label
text = "This is a\nmulti-line\nright-aligned text."
label = tk.Label(root, text=text, justify="right", width=40)
label.pack(pady=20)
root.mainloop()
Output in Windows:
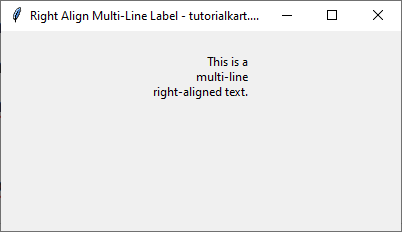
Conclusion
In this tutorial, we explored different ways to right-align text in a Tkinter Label:
- Using
anchor='e'
for single-line text. - Using
justify='right'
for multi-line text.
By aligning labels properly, you can improve the layout and readability of your Tkinter applications.