Right Align Text in Text Widget in Tkinter Python
In Tkinter, the Text
widget allows you to display and edit multi-line text. By default, text is left-aligned. However, you can right-align text using the tag_configure
method with the justify
option set to right
.
To right-align text in a Tkinter Text widget, follow these steps:
- Use the
Text
widget to create a multi-line text area. - Define a text tag using
text_widget.tag_configure("right", justify="right")
. - Insert the text into the widget and apply the tag using
text_widget.tag_add("right", "1.0", "end")
.
In this tutorial, we will go through examples using the tag_configure
method to right-align text in a Tkinter Text widget.
Examples
1. Right Aligning Text in a Tkinter Text Widget
In this example, we will create a Tkinter window with a Text widget and align the text to the right using the tag_configure
method.
We begin by creating a Tkinter window with a Text widget. We use the tag_configure
method to create a tag named "right"
that applies right alignment. Then, we insert text into the Text widget and apply the tag using tag_add
. This ensures that all text from the first character to the end of the text widget is aligned to the right.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Right Align Text - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Configure a tag for right alignment
text_widget.tag_configure("right", justify="right")
# Insert text and apply right alignment
text_widget.insert("1.0", "This text is right aligned in the Tkinter Text widget.")
text_widget.tag_add("right", "1.0", "end")
root.mainloop()
Output in Windows:
A Text widget appears in the Tkinter window with the given text aligned to the right side.
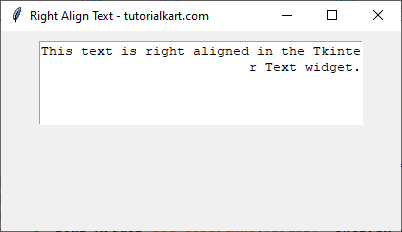
2. Right Aligning Multiple Lines of Text
In this example, we will add multiple lines of text and align them all to the right using a single tag.
We create a Text widget and apply a right-alignment tag named "right"
. Instead of a single line, we insert multiple lines of text using the insert
method. By applying the tag_add
method from the first character (1.0) to the end (“end”), all lines within the widget are right-aligned.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Right Align Multi-Line Text - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Configure a tag for right alignment
text_widget.tag_configure("right", justify="right")
# Insert multiple lines of text
text_widget.insert("1.0", "Line 1: Right aligned text\n")
text_widget.insert("2.0", "Line 2: Another right aligned line\n")
text_widget.insert("3.0", "Line 3: More right aligned text")
# Apply right alignment tag to all text
text_widget.tag_add("right", "1.0", "end")
root.mainloop()
Output in Windows:
A multi-line Text widget appears in the Tkinter window with all lines of text aligned to the right.
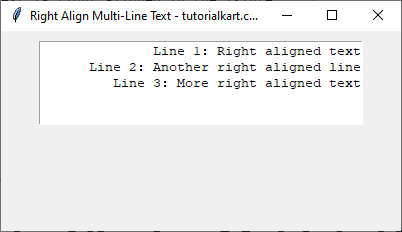
Conclusion
In this tutorial, we explored how to right-align text in Tkinter’s Text widget using the tag_configure
method with the justify="right"
option.