Set a Default Value for Entry Widget in Tkinter Python
In Tkinter, the Entry
widget is used to accept single-line text input from users. Sometimes, we need to set a default value in the Entry widget to provide a hint or pre-fill a value.
There are multiple ways to set a default value for an Entry widget in Tkinter:
- Using the
insert()
method - Using the
textvariable
option with aStringVar
In this tutorial, we will explore different methods to set a default value in the Tkinter Entry widget.
Examples
1. Setting Default Value for Entry Widget Using insert()
Method
We can use the insert()
method to insert text into the Entry widget at the start (index 0
).
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Entry Default Value - tutorialkart.com")
root.geometry("400x200")
# Create Entry widget
entry = tk.Entry(root, width=30)
entry.pack(pady=20)
# Insert default text
entry.insert(0, "Enter your name")
# Run the application
root.mainloop()
Output in Windows:
An Entry widget appears with the default text Enter your name
inside it.
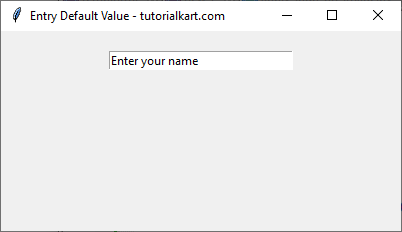
2. Setting Default Value for Entry Widget Using textvariable
with StringVar
Another way to set a default value in the Entry widget is by using a StringVar
variable.
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Entry Default Value - tutorialkart.com")
root.geometry("400x200")
# Define StringVar with default value
default_text = tk.StringVar()
default_text.set("Enter your email")
# Create Entry widget with textvariable
entry = tk.Entry(root, width=30, textvariable=default_text)
entry.pack(pady=20)
# Run the application
root.mainloop()
Output in Windows:
An Entry widget appears with the default text Enter your email
inside it.
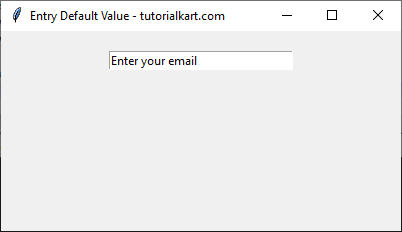
3. Setting Default Value and Clearing on Focus
We can also clear the default text when the user clicks inside the Entry widget.
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Entry Default Value - tutorialkart.com")
root.geometry("400x200")
def on_focus_in(event):
if entry.get() == "Enter password":
entry.delete(0, tk.END)
def on_focus_out(event):
if entry.get() == "":
entry.insert(0, "Enter password")
# Create Entry widget
entry = tk.Entry(root, width=30)
entry.pack(pady=20)
# Insert default text
entry.insert(0, "Enter password")
# Bind events for focus
entry.bind("<FocusIn>", on_focus_in)
entry.bind("<FocusOut>", on_focus_out)
# Run the application
root.mainloop()
Output in Windows:
An Entry widget appears with the default text Enter password
.
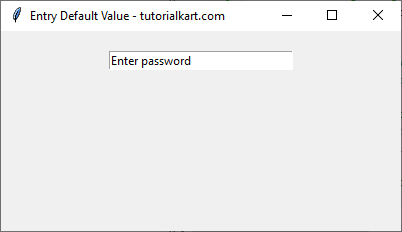
When clicked, the text disappears.
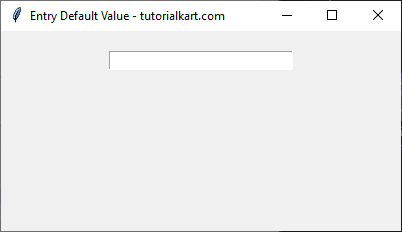
If left empty and clicked elsewhere, the default text reappears.
Conclusion
In this tutorial, we explored different methods to set a default value in the Tkinter Entry widget:
- Using the
insert()
method to insert text at index0
. - Using the
textvariable
option with aStringVar
variable. - Clearing the default value when clicked and restoring it if left empty.
By implementing these methods, you can improve the user experience in your Tkinter application.