Set a Default Value for Text Widget in Tkinter Python
In Tkinter, the Text
widget is used to display and edit multi-line text. By default, a Text
widget starts empty, but you can set a default value using the insert()
method.
The insert()
method allows you to pre-populate the Text
widget with a default value at a specific position. The syntax is:
text_widget.insert(index, default_text)
Here, index
defines the position where the text should be inserted. The value '1.0'
refers to the start of the text area (line 1, character 0).
In this tutorial, we will explore different ways to set a default value in a Text
widget using the insert()
method.
Examples
1. Setting a Default Value in a Text Widget
In this example, we create a simple Tkinter application where the Text
widget is pre-filled with a default message.
We define a Tkinter window with a Text
widget and insert a default value using insert()
. The default text is added at position '1.0'
to ensure it appears at the beginning of the widget.
main.py
import tkinter as tk
# Create the main application window
root = tk.Tk()
root.title("Text Widget Default Value - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=20)
# Insert default text
text_widget.insert("1.0", "Enter your text here...")
# Run the application
root.mainloop()
Output in Windows:
A text box appears with the default value “Enter your text here…” already filled in.
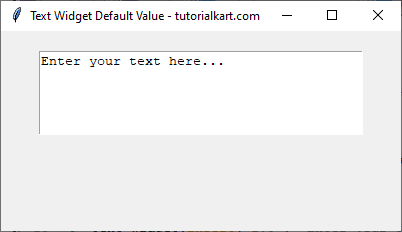
2. Setting a Default Value in Text Widget and Making It Read-Only
In this example, we set a default text in the Text
widget and make it read-only so users cannot edit the pre-filled content.
To achieve this, we first insert the default text, then set the widget’s state to DISABLED
to prevent user modifications.
main.py
import tkinter as tk
# Create the main application window
root = tk.Tk()
root.title("Read-Only Text Widget - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=20)
# Insert default text
text_widget.insert("1.0", "This text is read-only.")
# Make the text widget read-only
text_widget.config(state=tk.DISABLED)
# Run the application
root.mainloop()
Output in Windows:
A text box appears with the default value “This text is read-only.” Users cannot modify the content as the widget is disabled.
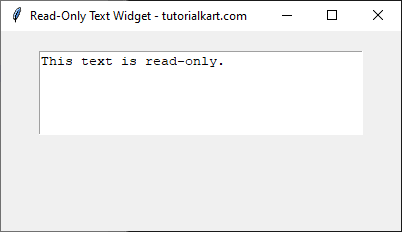
Conclusion
In this tutorial, we explored how to set a default value for a Text
widget in Tkinter using the insert()
method. We also learned how to make the text read-only using the config(state=tk.DISABLED)
option.
- Use
insert("1.0", "default text")
to pre-fill theText
widget. - Set
state=tk.DISABLED
to prevent users from modifying the content.