Set a Value in Entry Widget in Tkinter Python
In Tkinter, the Entry
widget is used to take input from the user. You can set a default value in the Entry
field using the insert()
method or by using the StringVar
class.
Setting a default value in an Entry widget is useful when you want to prepopulate the input field with commonly used values or placeholders.
In this tutorial, we will go through examples to set values in an Entry Widget in Tkinter Python.
Examples
1. Setting a Value in Entry widget Using insert() Method
In this example, we will use the insert()
method to insert a default value in an Entry widget.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Tkinter Entry Example - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget
entry = tk.Entry(root)
entry.pack(pady=20)
# Set a default value
entry.insert(0, "Enter your name")
root.mainloop()
Output in Windows:
An Entry field is displayed with the text “Enter your name” already filled in.
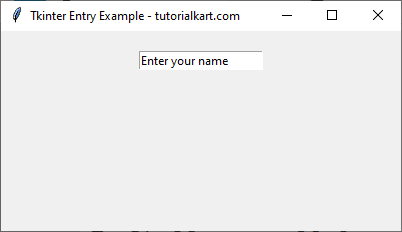
2. Setting a Value Using StringVar() in Entry Widget
Another way to set a default value is by using the StringVar
class, which allows you to dynamically control the value of an Entry widget.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Tkinter Entry Example - tutorialkart.com")
root.geometry("400x200")
# Define a StringVar
entry_text = tk.StringVar()
entry_text.set("Default Value")
# Create an Entry widget with StringVar
entry = tk.Entry(root, textvariable=entry_text)
entry.pack(pady=20)
root.mainloop()
Output in Windows:
An Entry field is displayed with the text “Default Value” already filled in.
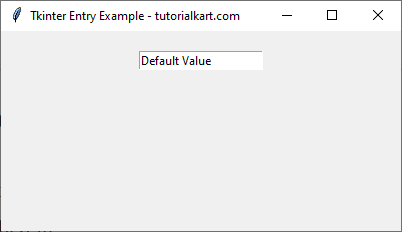
3. Updating the Entry Value on Button Click
In this example, we will update the Entry widget’s value dynamically when a button is clicked.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Tkinter Entry Example - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget
entry = tk.Entry(root)
entry.pack(pady=10)
# Function to update Entry value
def update_value():
entry.delete(0, tk.END) # Clear existing text
entry.insert(0, "Updated Value")
# Create a button to update Entry value
button = tk.Button(root, text="Set Value", command=update_value)
button.pack(pady=10)
root.mainloop()
Output in Windows:
An Entry field appears empty initially.
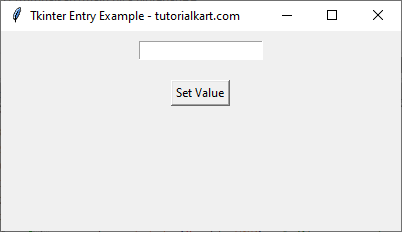
When the button is clicked, “Updated Value” appears in the Entry field.
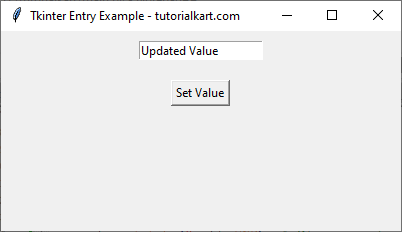
Conclusion
In this tutorial, we explored how to set a value in an Entry widget in Tkinter using:
- The
insert()
method - The
StringVar()
class - Updating the Entry value dynamically with a button click
Using these methods, you can prepopulate or update input fields dynamically in your Tkinter applications.