Set a Value in Text Widget in Tkinter Python
In Tkinter, the Text
widget is used to display multi-line text and allows users to edit or modify its content. To set a value (text) inside a Text
widget, we use the insert()
method, which allows inserting text at a specific position.
</>
Copy
insert(index, text)
The insert()
method takes two parameters:
- index: Specifies the position where the text should be inserted. Common values are:
'1.0'
– Start of the text widget.'end'
– Appends text to the end.
- text: The string to insert into the Text widget.
Examples
1. Setting a Default Value in a Text Widget
In this example, we create a Text widget and insert a default message at the beginning.
main.py
</>
Copy
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Set Text in Tkinter - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Insert default text
text_widget.insert("1.0", "Welcome to Tkinter Text Widget!")
root.mainloop()
Output in Windows:
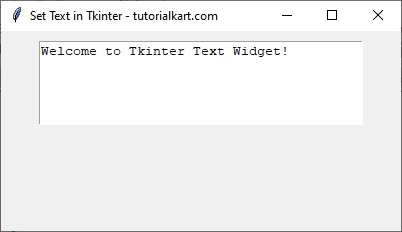
2. Setting a Value Dynamically Using a Button
In this example, we insert text into the Text widget when the button is clicked.
main.py
</>
Copy
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Dynamic Text Insertion - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Define function to insert text
def insert_text():
text_widget.insert("1.0", "Hello, Tkinter!\n")
# Create a button to insert text
btn_insert = tk.Button(root, text="Insert Text", command=insert_text)
btn_insert.pack(pady=5)
root.mainloop()
Output in Windows:
The following is the initial screen when you run the program.
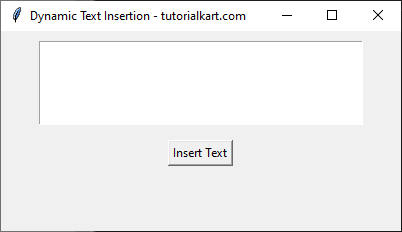
Click on the Insert Text button.
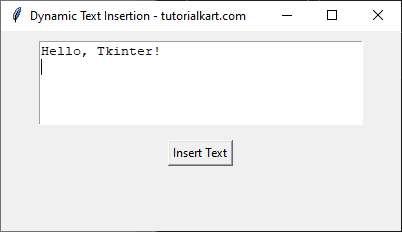
The string Hello, Tkinter!
is written to the Text field.
Conclusion
In this tutorial, we explored how to set text inside a Tkinter Text
widget using the insert()
method. We covered:
- Setting a default value in a Text widget.
- Using a button to dynamically insert text.