Set Auto-scroll for Text Widget in Tkinter Python
In Tkinter, the Text
widget is used for multi-line text input. However, when the content inside the Text widget grows beyond its visible area, it requires a scrollbar to navigate. Auto-scrolling ensures that when new content is added, the Text widget automatically scrolls to the bottom.
To implement auto-scroll in a Text widget, we use the yview
method to keep the scrollbar and text view updated as new content is added.
In this tutorial, we will explore how to enable auto-scrolling in a Tkinter Text Widget using different approaches.
Examples
1. Auto-scroll Text Widget with a Scrollbar
In this example, we create a Text widget with a scrollbar. When new text is added, the widget automatically scrolls to the bottom.
import tkinter as tk
from tkinter import scrolledtext
# Create the main window
root = tk.Tk()
root.title("Auto-scroll Text Widget - tutorialkart.com")
root.geometry("400x500")
# Create a frame to hold Text widget and scrollbar
frame = tk.Frame(root)
frame.pack(fill=tk.BOTH, expand=True)
# Create a Scrollbar
scrollbar = tk.Scrollbar(frame)
scrollbar.pack(side=tk.RIGHT, fill=tk.Y)
# Create a Text widget
text_widget = tk.Text(frame, wrap=tk.WORD, yscrollcommand=scrollbar.set)
text_widget.pack(fill=tk.BOTH, expand=True)
# Attach scrollbar to Text widget
scrollbar.config(command=text_widget.yview)
# Function to insert text and auto-scroll
def add_text():
text_widget.insert(tk.END, "New line added\n")
text_widget.yview(tk.END) # Auto-scroll to the bottom
# Create a button to add text
btn = tk.Button(root, text="Add Text", command=add_text)
btn.pack()
root.mainloop()
Output in Windows:
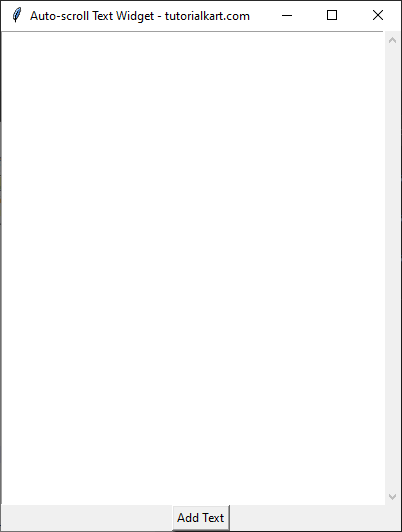
Clicking the “Add Text” button appends a new line to the Text widget and automatically scrolls to the bottom.
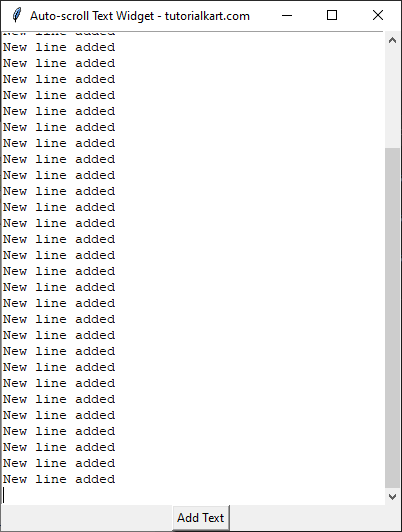
2. Auto-scroll Text Widget with Realtime Updates
In this example, we simulate real-time text updates in the Text widget. A function continuously adds text at intervals, ensuring the latest content is always visible.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Auto-scroll Text Widget - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget with a scrollbar
frame = tk.Frame(root)
frame.pack(fill=tk.BOTH, expand=True)
scrollbar = tk.Scrollbar(frame)
scrollbar.pack(side=tk.RIGHT, fill=tk.Y)
text_widget = tk.Text(frame, wrap=tk.WORD, yscrollcommand=scrollbar.set)
text_widget.pack(fill=tk.BOTH, expand=True)
scrollbar.config(command=text_widget.yview)
# Function to add text at intervals
def update_text():
text_widget.insert(tk.END, "Updating...\n")
text_widget.yview(tk.END) # Auto-scroll
root.after(1000, update_text) # Repeat every second
# Start updating text
update_text()
root.mainloop()
Output in Windows:
The Text widget updates in real-time, automatically scrolling as new text is appended.
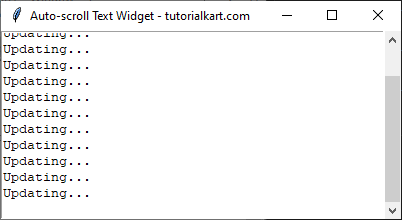
Conclusion
In this tutorial, we explored how to enable auto-scrolling in a Tkinter Text widget using the yview
method. Auto-scroll can be useful for real-time logging, chat applications, and dynamically updating text fields.
- We used a scrollbar to allow manual scrolling.
- We implemented automatic scrolling using
yview(tk.END)
. - We created a real-time updating text widget that continuously appends new text.