Set Background Color for Entry Widget in Tkinter Python
In Tkinter, the bg
(background) option of an Entry
widget allows you to set the background color. This is useful for improving the visual appeal and user experience of input fields.
To set a specific background color for an Entry widget, pass the bg
parameter with a valid color name or hex code while creating the widget.
In this tutorial, we will explore different ways to set the background color of a Tkinter Entry Widget using color names and hex codes.
Examples
1. Setting Background Color for Entry Widget Using Standard Color Names
In this example, we will set the background color of an Entry widget using a standard color name like "lightblue"
.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Entry Background Color - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget with a light blue background
entry = tk.Entry(root, bg="lightblue", font=("Arial", 14))
entry.pack(pady=20)
root.mainloop()
Output in Windows:
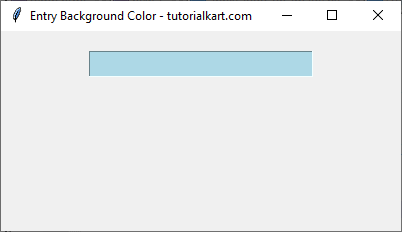
2. Setting Background Color for Entry Widget Using Hex Code (#rrggbb)
In this example, we will set the background color of an Entry widget using an 8-bit hex code (#ffeb3b
for yellow).
import tkinter as tk
root = tk.Tk()
root.title("Entry Background Color - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget with a yellow background
entry = tk.Entry(root, bg="#ffeb3b", font=("Arial", 14))
entry.pack(pady=20)
root.mainloop()
Output in Windows:
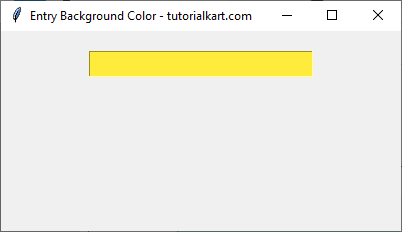
3. Setting Background Color for Entry Widget Using 4-bit Per Color Hex Code (#rgb)
In this example, we will use a 4-bit hex code #faa
(light red) as the background color.
import tkinter as tk
root = tk.Tk()
root.title("Entry Background Color - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget with a light red background
entry = tk.Entry(root, bg="#faa", font=("Arial", 14))
entry.pack(pady=20)
root.mainloop()
Output in Windows:
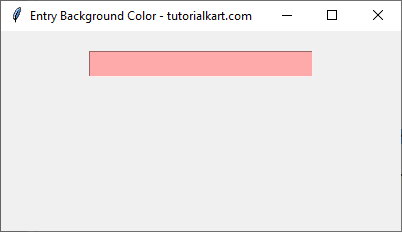
4. Changing Background Color Dynamically for Entry Widget
In this example, we will allow the user to change the background color of the Entry widget dynamically.
import tkinter as tk
root = tk.Tk()
root.title("Entry Background Color - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget with an initial background color
entry = tk.Entry(root, bg="white", font=("Arial", 14))
entry.pack(pady=10)
# Function to change background color
def change_color():
entry.config(bg="lightgreen")
# Create a button to change the color
button = tk.Button(root, text="Change Color", command=change_color)
button.pack(pady=10)
root.mainloop()
Output in Windows:
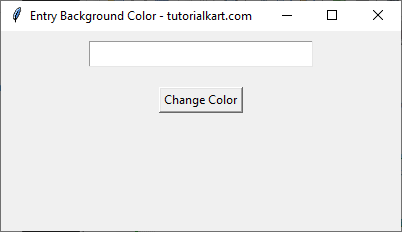
Click on Change Color button.
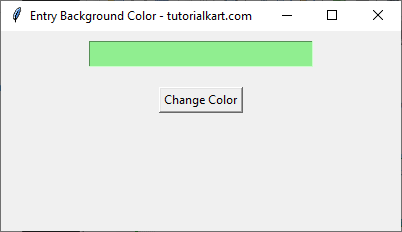
Conclusion
In this tutorial, we explored how to set the background color of an Entry
widget in Tkinter using:
- Standard color names (e.g.,
"lightblue"
) - 8-bit hex codes (e.g.,
#ffeb3b
) - 4-bit hex codes (e.g.,
#faa
) - Dynamic color change using the
config()
method