Set Background Color for Label in Tkinter Python
In Tkinter, the bg
(background) option of a Label widget allows you to set the background color of the label. The color can be specified in different formats, including:
- 4-bit per color hex code (
#rgb
) - 8-bit per color hex code (
#rrggbb
) - 12-bit per color hex code (
#rrrgggbbb
) - Standard color names like
'red'
,'blue'
, and'yellow'
In this tutorial, we will explore different ways to set the background color of a Label widget in a Tkinter Label.
Examples
1. Setting Background Color Using 4-bit Per Color Hex Code
In this example, we set the label background color using a 4-bit per color hex code (#rgb
format).
import tkinter as tk
root = tk.Tk()
root.title("Label Background Color Example - tutorialkart.com")
root.geometry("400x200")
# Create a label with a 4-bit per color hex background
label = tk.Label(root, text="Hello, Tkinter!", bg="#f00", fg="white", font=("Arial", 14))
label.pack(pady=20)
root.mainloop()
Output in Windows:
A label with a red background and white text is displayed.
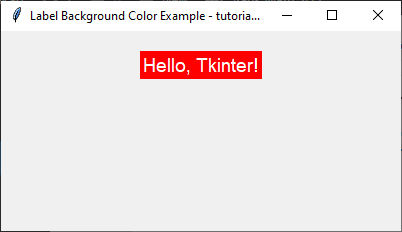
2. Setting Background Color Using 8-bit Per Color Hex Code
In this example, we set the background color using an 8-bit per color hex code (#rrggbb
format).
import tkinter as tk
root = tk.Tk()
root.title("Label Background Color Example - tutorialkart.com")
root.geometry("400x200")
# Create a label with an 8-bit per color hex background
label = tk.Label(root, text="Welcome to Tkinter!", bg="#3498db", fg="white", font=("Arial", 14))
label.pack(pady=20)
root.mainloop()
Output in Windows:
A label with a blue background and white text is displayed.
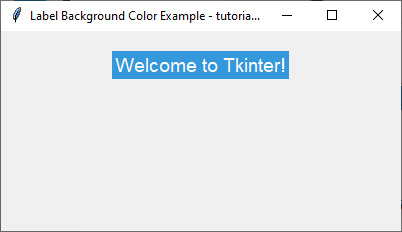
3. Setting Background Color Using 12-bit Per Color Hex Code
In this example, we set the background color using a 12-bit per color hex code (#rrrgggbbb
format).
import tkinter as tk
root = tk.Tk()
root.title("Label Background Color Example - tutorialkart.com")
root.geometry("400x200")
# Create a label with a 12-bit per color hex background
label = tk.Label(root, text="Tkinter Label", bg="#fa8aba53a", fg="white", font=("Arial", 14))
label.pack(pady=20)
root.mainloop()
Output in Windows:
A label with a custom shade background and white text is displayed.
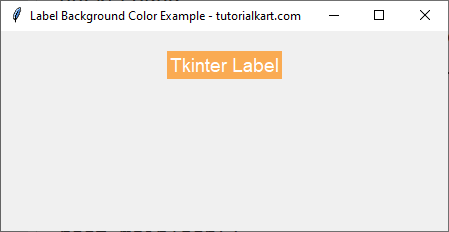
4. Setting Background Color Using Standard Color Names
In this example, we set the background color using standard color names like 'yellow'
, 'green'
, and 'purple'
.
import tkinter as tk
root = tk.Tk()
root.title("Label Background Color Example - tutorialkart.com")
root.geometry("400x200")
# Create labels with standard color names
label1 = tk.Label(root, text="Yellow Background", bg="yellow", fg="black", font=("Arial", 14))
label1.pack(pady=5)
label2 = tk.Label(root, text="Green Background", bg="green", fg="white", font=("Arial", 14))
label2.pack(pady=5)
label3 = tk.Label(root, text="Purple Background", bg="purple", fg="white", font=("Arial", 14))
label3.pack(pady=5)
root.mainloop()
Output in Windows:
Three labels are displayed with yellow, green, and purple backgrounds, respectively.
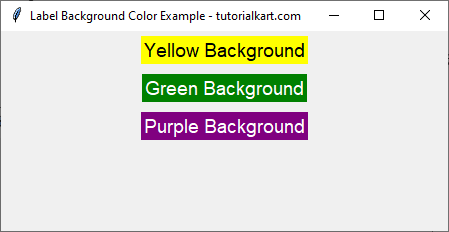
Conclusion
In this tutorial, we explored how to use the bg
option in Tkinter’s Label widget to control the background color. The color can be set using:
- 4-bit per color hex codes (
#rgb
) - 8-bit per color hex codes (
#rrggbb
) - 12-bit per color hex codes (
#rrrgggbbb
) - Standard color names (
'yellow'
,'green'
, etc.)
By customizing the background color, you can enhance the visual appeal of labels in your Tkinter application.