Background Color for Text Widget in Tkinter Python
In Tkinter, the Text
widget is used for multi-line text input. To change the background color of a Text widget, we use the bg
option. This option accepts color values in different formats, including standard color names, hex codes, and RGB values.
In this tutorial, we will explore different ways to set the background color of a Tkinter Text widget with practical examples.
Examples
1. Setting Background Color for Text Widget Using a Standard Color Name
In this example, we create a Text
widget with a yellow background using the bg
parameter.
The program creates a Tkinter window titled tutorialkart.com with a size of 400x200
. We define a Text
widget named text_widget
and specify bg="yellow"
to change the background color to yellow. Finally, we pack the widget to make it visible in the window.
main.py
import tkinter as tk
# Create the main application window
root = tk.Tk()
root.title("tutorialkart.com - Text Widget Background")
root.geometry("400x200")
# Create a Text widget with a yellow background
text_widget = tk.Text(root, height=5, width=40, bg="yellow")
text_widget.pack(pady=10)
# Run the Tkinter event loop
root.mainloop()
Output in Windows:
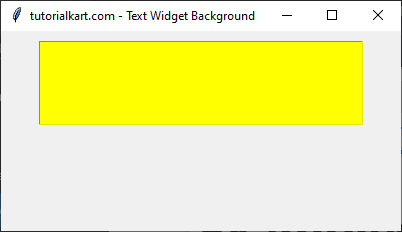
You may enter some text.
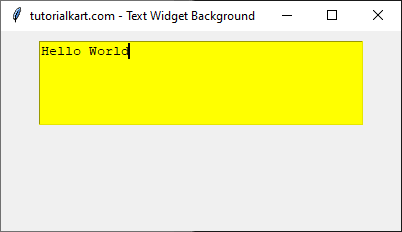
2. Setting Background Color for Text Widget Using Hex Code
In this example, we use a hex color code (#87CEEB
) to set a light blue background color for the Text widget.
The program initializes a Tkinter window named tutorialkart.com with dimensions 400x200
. We create a Text
widget called text_widget
with bg="#87CEEB"
to apply the light blue color. The height
and width
properties control the size of the text area, and we use pack()
to display the widget.
main.py
import tkinter as tk
# Create the main application window
root = tk.Tk()
root.title("tutorialkart.com - Text Widget Background")
root.geometry("400x200")
# Create a Text widget with a light blue background using a hex code
text_widget = tk.Text(root, height=5, width=40, bg="#87CEEB")
text_widget.pack(pady=10)
# Run the Tkinter event loop
root.mainloop()
Output in Windows:
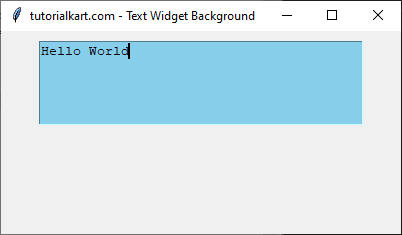
Conclusion
In this tutorial, we explored how to change the background color of a Tkinter Text widget using the bg
option. We demonstrated two approaches:
- Using a standard color name (e.g.,
"yellow"
). - Using a hex color code (e.g.,
"#87CEEB"
for light blue).