Set Background Image for Label in Tkinter Python
In Tkinter, you can set a background image for a Label widget using the PhotoImage
class. The image is placed as the background, and you can overlay text on it using the text
parameter of the Label widget.
To set a background image for a Label in Tkinter, follow these steps:
- Load the image using
PhotoImage
. - Create a
Label
widget and set theimage
parameter to the loaded image. - Use the
compound
parameter to display text on the image.
In this tutorial, we will go through multiple examples of setting a background image in a Tkinter Label.
Examples
1. Setting a Background Image for Label
In this example, we will load an image and set it as the background of a Label widget.
import tkinter as tk
from tkinter import PhotoImage
root = tk.Tk()
root.title("tutorialkart.com")
root.geometry("400x200")
# Load the image
bg_image = PhotoImage(file="background-image.png")
# Create a label with the image as background
label = tk.Label(root, image=bg_image)
label.pack(fill="both", expand=True)
root.mainloop()
Output in Windows:
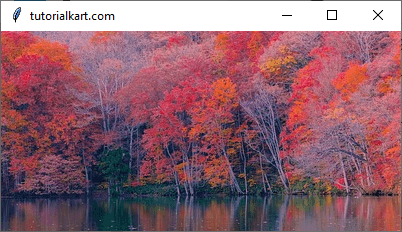
2. Displaying Text on the Background Image
In this example, we will overlay text on the background image using the text
and compound
parameters.
import tkinter as tk
from tkinter import PhotoImage
root = tk.Tk()
root.title("tutorialkart.com")
root.geometry("400x200")
# Load the image
bg_image = PhotoImage(file="background-image.png")
# Create a label with the image as background and add text
label = tk.Label(root, image=bg_image, text="Welcome to Tkinter!", font=("Arial", 14, "bold"), fg="white", compound="center")
label.pack(fill="both", expand=True)
root.mainloop()
Output in Windows:
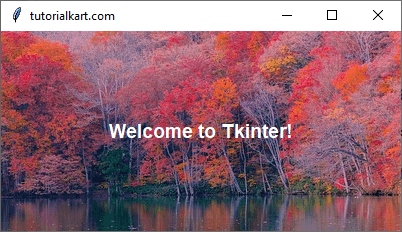
3. Adjusting Text Position on the Background Image
We can modify the text position by using the anchor
parameter.
import tkinter as tk
from tkinter import PhotoImage
root = tk.Tk()
root.title("tutorialkart.com")
root.geometry("400x200")
# Load the image
bg_image = PhotoImage(file="background-image.png")
# Create a label with text positioned at the bottom right
label = tk.Label(root, image=bg_image, text="Tkinter Example", font=("Arial", 14, "bold"), fg="yellow", compound="center", anchor="se")
label.pack(fill="both", expand=True)
root.mainloop()
Output in Windows:
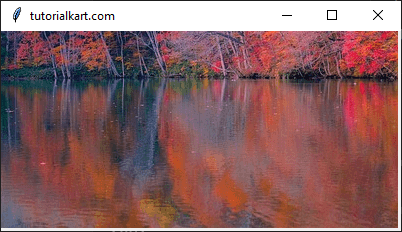
4. Setting a Resizable Background Image
To make the background image resizable with the window, use the Canvas
widget with create_image()
.
import tkinter as tk
from PIL import Image, ImageTk
root = tk.Tk()
root.title("tutorialkart.com")
root.geometry("400x200")
# Load the image and resize it
image = Image.open("background-image.png")
image = image.resize((400, 200), Image.LANCZOS)
bg_image = ImageTk.PhotoImage(image)
# Create a canvas and set the image as background
canvas = tk.Canvas(root, width=400, height=200)
canvas.pack(fill="both", expand=True)
canvas.create_image(0, 0, image=bg_image, anchor="nw")
# Add text to the canvas
canvas.create_text(200, 100, text="Tkinter Resizable Background", font=("Arial", 14, "bold"), fill="white")
root.mainloop()
Output in Windows:
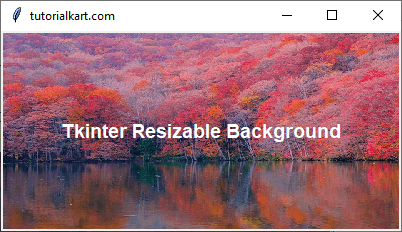
Conclusion
In this tutorial, we explored multiple methods to set a background image for a Label in Tkinter. We covered:
- Setting a static background image.
- Overlaying text on the background image.
- Adjusting text position using
anchor
. - Creating a resizable background image using
Canvas
.
Using these techniques, you can customize labels in your Tkinter applications with visually appealing backgrounds.