Set Bold Font for Entry Widget in Tkinter Python
In Tkinter, the Entry
widget is used to create input fields where users can type text. You can set the font style, including bold formatting, using the font
option.
The font
parameter takes a tuple containing three values:
- Font family (e.g.,
"Arial"
,"Times New Roman"
) - Font size (e.g.,
12
,16
) - Font style (e.g.,
"bold"
,"italic"
)
In this tutorial, we will go through multiple examples to set a bold font for the Entry
widget in a Tkinter Entry widget.
Examples
1. Setting Bold Font for Entry Widget
In this example, we will create a Tkinter window with an Entry widget that uses a bold font.
</>
Copy
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("tutorialkart.com - Bold Entry Example")
root.geometry("400x200")
# Create an Entry widget with a bold font
entry = tk.Entry(root, font=("Arial", 14, "bold"))
entry.pack(pady=20)
# Run the application
root.mainloop()
Output in Windows:
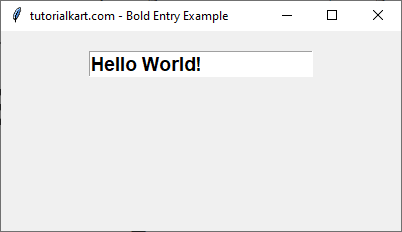
2. Setting Bold and Italic Font for Entry Widget
In this example, we will apply both bold and italic styles to the text inside the Entry widget.
</>
Copy
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("tutorialkart.com - Bold & Italic Entry Example")
root.geometry("400x200")
# Create an Entry widget with bold and italic font
entry = tk.Entry(root, font=("Times New Roman", 16, "bold italic"))
entry.pack(pady=20)
# Run the application
root.mainloop()
Output in Windows:
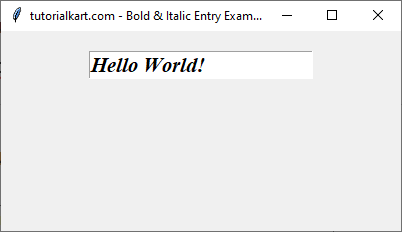
3. Setting Bold Font and Custom Font Size for Entry widget
Here, we will create an Entry widget with a bold font and a larger font size.
</>
Copy
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("tutorialkart.com - Large Bold Entry")
root.geometry("400x200")
# Create an Entry widget with a large bold font
entry = tk.Entry(root, font=("Verdana", 18, "bold"))
entry.pack(pady=20)
# Run the application
root.mainloop()
Output in Windows:
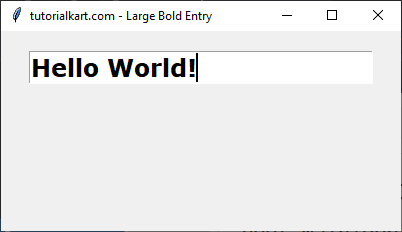
Conclusion
In this tutorial, we explored how to use the font
option in Tkinter’s Entry widget to set bold text. The font formatting includes:
- Applying bold font using
("Arial", 14, "bold")
- Combining bold and italic styles with
("Times New Roman", 16, "bold italic")
- Increasing the font size for better readability using
("Verdana", 18, "bold")