Bold Font for Text Widget in Tkinter Python
In Tkinter, the Text
widget is used to display and edit multiple lines of text. You can set a bold font for the text inside a Text
widget by specifying a font style that includes the weight='bold'
attribute.
To apply bold text in a Tkinter Text
widget, you need to:
- Use the
font
option while creating the Text widget. - Apply a tag to specific text using
text_widget.tag_add()
. - Configure the tag with a bold font using
text_widget.tag_config()
.
In this tutorial, we will go through examples using different methods to set bold font in a Tkinter Text Widget.
Examples
1. Setting Bold Font for Entire Text in a Text Widget
In this example, we will create a Tkinter Text
widget and set the entire text content to a bold font.
We are using the font=("Arial", 12, "bold")
attribute to set the text inside the Text widget to bold. The Text
widget is initialized with the variable text_widget
, and the insert()
method is used to add default text.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Bold Text in Tkinter - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget with bold font
text_widget = tk.Text(root, font=("Arial", 12, "bold"))
text_widget.pack(pady=10)
# Insert sample text
text_widget.insert(tk.END, "This is bold text inside a Text widget.")
# Run the application
root.mainloop()
Output in Windows:
The text inside the Text widget appears in bold using the Arial font with a size of 12.
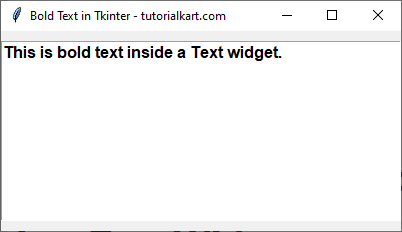
2. Setting Bold Font for Specific Text Using Tags in Text Widget
In this example, we will make only a specific part of the text inside a Text
widget bold by using tags.
We are inserting two lines of text into the Text
widget and then applying the bold_tag
to the second line using text_widget.tag_add()
. The tag is configured using text_widget.tag_config()
with the font set to bold.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Bold Text with Tags - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, font=("Arial", 12))
text_widget.pack(pady=10)
# Insert sample text
text_widget.insert(tk.END, "This is normal text.\n")
text_widget.insert(tk.END, "This text will be bold.")
# Define a tag for bold text
text_widget.tag_config("bold_tag", font=("Arial", 12, "bold"))
# Apply the tag to the second line
text_widget.tag_add("bold_tag", "2.0", "2.end")
# Run the application
root.mainloop()
Output in Windows:
The first line appears in normal font, while the second line is displayed in bold.
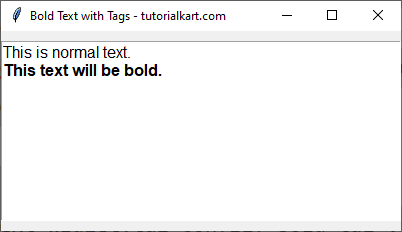
Conclusion
In this tutorial, we explored different ways to set bold font for a Text
widget in Tkinter:
- Setting bold font for the entire text using
font=("Arial", 12, "bold")
. - Applying bold font to specific parts of text using
text_widget.tag_add()
andtext_widget.tag_config()
.