Set Bold Text for Button in Tkinter
In Tkinter, the font
attribute of the Button widget allows you to customize the appearance of the text, including making it bold. The font
attribute accepts a tuple specifying the font family, size, and style.
To make the text bold, you can pass "bold"
as a style parameter in the font
attribute:
tk.Button(window, text="Bold Button", font=("Arial", 14, "bold"))
In this tutorial, we will go through examples using the font
attribute to apply bold text in a Tkinter Button.
Examples
1. Setting Bold Text for Button
In this example, we will create a Tkinter button with bold text using the font
attribute.
import tkinter as tk
root = tk.Tk()
root.title("Bold Text in Button")
root.geometry("400x200")
# Create a button with bold text
button = tk.Button(root, text="Bold Button", font=("Arial", 14, "bold"))
button.pack(pady=10)
root.mainloop()
Screenshot in Windows
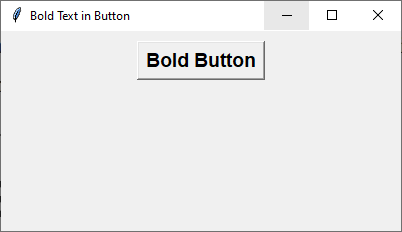
2. Setting Bold and Italic Text for Button
To apply both bold and italic styles, you can pass "bold italic"
in the font
attribute.
import tkinter as tk
root = tk.Tk()
root.title("Bold and Italic Button")
root.geometry("400x200")
# Create a button with bold and italic text
button = tk.Button(root, text="Bold & Italic Button", font=("Verdana", 14, "bold italic"))
button.pack(pady=10)
root.mainloop()
Output:
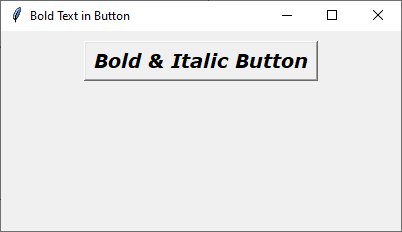
3. Setting Bold Text with Different Font Families
You can also change the font family while making the text bold. Here’s an example using “Courier New”.
import tkinter as tk
root = tk.Tk()
root.title("Different Font Style in Button")
root.geometry("400x200")
# Create buttons with different font families
btn_courier = tk.Button(root, text="Courier Bold", font=("Courier New", 12, "bold"))
btn_courier.pack(pady=5)
btn_tahoma = tk.Button(root, text="Tahoma Bold", font=("Tahoma", 14, "bold"))
btn_tahoma.pack(pady=5)
root.mainloop()
Output:
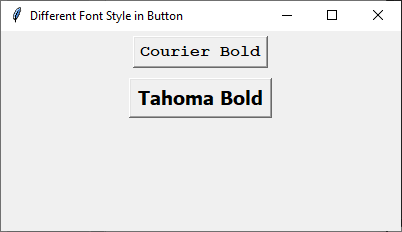
Conclusion
In this tutorial, we explored how to use the font
attribute in Tkinter’s Button widget to set bold text. You can:
- Use
font=("Arial", 14, "bold")
to make the text bold. - Use
"bold italic"
for both bold and italic styles. - Change the font family to “Courier New”, “Verdana”, or “Tahoma” for different looks.
By customizing the text style, you can improve the visual appearance of buttons in your Tkinter application.