Set Bold Text for Label in Tkinter Python
To make the text bold for Label in Tkinter, we can use the font
option by specifying a font family, size, and the style as 'bold'
.
The font format follows this pattern:
</>
Copy
tk.Label(root, text="Bold Text", font=("Arial", 14, "bold"))
In this tutorial, we will go through multiple examples demonstrating how to set bold text for a Tkinter Label.
Examples
1. Setting Bold Text for Label
In this example, we create a Tkinter label with bold text using the font
option.
main.py
</>
Copy
import tkinter as tk
root = tk.Tk()
root.title("Bold Label Example - tutorialkart.com")
root.geometry("400x200")
# Create a bold text label
label = tk.Label(root, text="This is Bold Text", font=("Arial", 14, "bold"))
label.pack(pady=20)
root.mainloop()
Output in Windows:
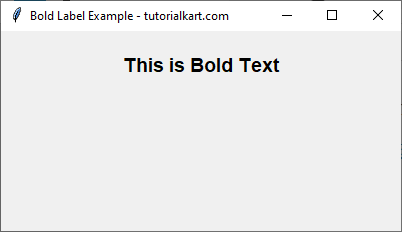
2. Using Different Font Families for Bold Text
We can use different font families such as Times New Roman
and Helvetica
to display bold text.
main.py
</>
Copy
import tkinter as tk
root = tk.Tk()
root.title("Bold Font Example - tutorialkart.com")
root.geometry("400x200")
# Create labels with different font families
label1 = tk.Label(root, text="Bold - Times New Roman", font=("Times New Roman", 16, "bold"))
label1.pack(pady=5)
label2 = tk.Label(root, text="Bold - Helvetica", font=("Helvetica", 16, "bold"))
label2.pack(pady=5)
root.mainloop()
Output in Windows:
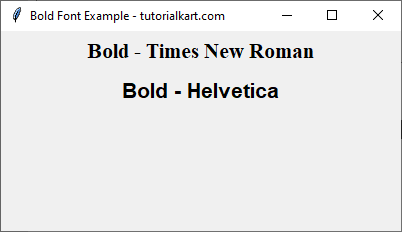
3. Setting Bold and Italic Text for Label
We can combine both bold
and italic
styles in a single label using ("FontName", size, "bold italic")
.
main.py
</>
Copy
import tkinter as tk
root = tk.Tk()
root.title("Bold Italic Label Example - tutorialkart.com")
root.geometry("400x200")
# Create a label with bold and italic text
label = tk.Label(root, text="Bold and Italic Text", font=("Arial", 16, "bold italic"))
label.pack(pady=20)
root.mainloop()
Output in Windows:
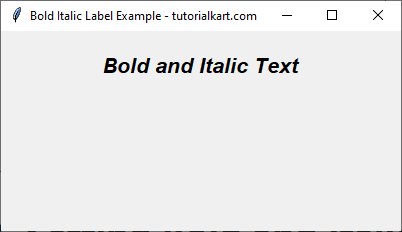
Conclusion
In this tutorial, we explored how to set bold text for the Label
widget in Tkinter using the font
option. We covered:
- Setting a simple bold font using
font=("Arial", 14, "bold")
. - Using different font families such as
Times New Roman
andHelvetica
. - Combining
bold
anditalic
styles.