How to Set Button Border Color in Tkinter Python
In Tkinter, the Button widget does not have a direct option to set the border color. However, you can achieve this effect by placing the Button inside a Frame
with a specified background color, effectively creating a border.
To set a border color for a button in Tkinter, follow these steps:
- Create a
Frame
widget with the desired border color. - Set the
Frame
’sbackground
color to match the desired border color. - Place the Button inside the
Frame
with proper padding.
In this tutorial, we will explore different ways to set a border color for a Tkinter Button using the Frame
widget.
Examples
1. Setting a Red Border Around a Button
In this example, we create a Button inside a Frame with a red border.
import tkinter as tk
root = tk.Tk()
root.title("Button Border Color Example - tutorialkart.com")
root.geometry("400x200")
# Create a Frame to act as a border
frame = tk.Frame(root, bg="red", padx=3, pady=3)
frame.pack(pady=20)
# Create a Button inside the Frame
button = tk.Button(frame, text="Click Me", bg="white")
button.pack()
root.mainloop()
Output:
A button with a red border is displayed.
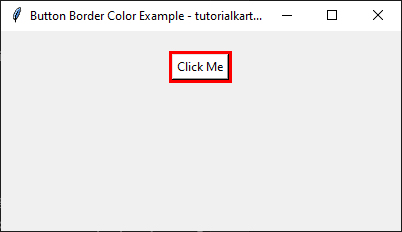
2. Setting a Blue Border Around a Button
This example sets a blue border around the Button.
import tkinter as tk
root = tk.Tk()
root.title("Button Border Color Example - tutorialkart.com")
root.geometry("400x200")
# Create a Frame with a blue border
frame = tk.Frame(root, bg="blue", padx=4, pady=4)
frame.pack(pady=20)
# Create a Button inside the Frame
button = tk.Button(frame, text="Click Me", bg="white")
button.pack()
root.mainloop()
Output:
A button with a blue border is displayed.
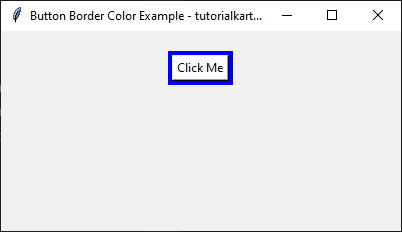
3. Creating a Thick Green Border
We increase the padding in the Frame to create a thick green border.
import tkinter as tk
root = tk.Tk()
root.title("Button Border Color Example - tutorialkart.com")
root.geometry("400x200")
# Create a Frame with a thick green border
frame = tk.Frame(root, bg="green", padx=10, pady=10)
frame.pack(pady=20)
# Create a Button inside the Frame
button = tk.Button(frame, text="Click Me", bg="white")
button.pack()
root.mainloop()
Output:
A button with a thick green border is displayed.
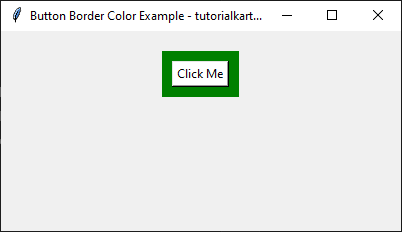
Conclusion
In this tutorial, we explored how to set a border color for Tkinter buttons using the Frame
widget. The key takeaways include:
- Placing the Button inside a Frame allows customization of the border color.
- Using
padx
andpady
increases the thickness of the border. - Setting a
relief
property can enhance the border effect.
By applying these techniques, you can create visually appealing buttons in your Tkinter application.