How to Set Button Color in Tkinter
In Tkinter, you can set the background and foreground (text) color of a Button using the bg
and fg
options, respectively. The background color changes the button’s surface, while the foreground color changes the text color.
The color can be specified in different formats:
- Hex color codes (e.g.,
#ff0000
,#00ff00
) - Standard color names (e.g.,
'red'
,'blue'
) - RGB values in the format
'rgb(r,g,b)'
(not directly supported but can be converted usingf'#{r:02x}{g:02x}{b:02x}'
)
In this tutorial, we will go through multiple examples using the bg
and fg
options to modify button colors in Tkinter.
Examples
1. Setting Button Background and Text Color Using Color Names
In this example, we create buttons with different background and text colors using standard color names.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Button Color Example - tutorialkart.com")
root.geometry("400x200")
# Create buttons with different background and text colors
btn_red = tk.Button(root, text="Red Button", bg="red", fg="white")
btn_red.pack(pady=5)
btn_green = tk.Button(root, text="Green Button", bg="green", fg="black")
btn_green.pack(pady=5)
btn_blue = tk.Button(root, text="Blue Button", bg="blue", fg="yellow")
btn_blue.pack(pady=5)
root.mainloop()
Output in Windows:
Three buttons appear with red, green, and blue backgrounds and corresponding text colors.
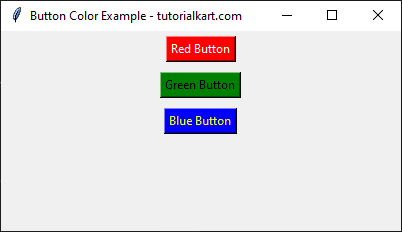
2. Setting Button Color Using Hex Codes
In this example, we set the button colors using hex codes for more precise color customization.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Hex Color Button Example - tutorialkart.com")
root.geometry("400x200")
# Create buttons with hex color codes
btn_orange = tk.Button(root, text="Orange Button", bg="#ff853a", fg="#000000")
btn_orange.pack(pady=5)
btn_purple = tk.Button(root, text="Purple Button", bg="#800080", fg="#ffffff")
btn_purple.pack(pady=5)
btn_cyan = tk.Button(root, text="Cyan Button", bg="#00ffff", fg="#000000")
btn_cyan.pack(pady=5)
root.mainloop()
Output in Windows:
Three buttons appear with orange, purple, and cyan backgrounds and corresponding text colors.
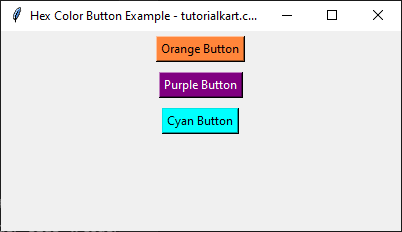
3. Setting Button Color Using RGB Values
Although Tkinter does not support direct RGB values, we can convert them to hex codes dynamically.
main.py
import tkinter as tk
root = tk.Tk()
root.title("RGB Color Button Example - tutorialkart.com")
root.geometry("400x200")
# Convert RGB to Hex
def rgb_to_hex(r, g, b):
return f'#{r:02x}{g:02x}{b:02x}'
# Create buttons with RGB color converted to hex
btn_yellow = tk.Button(root, text="Yellow Button", bg=rgb_to_hex(255, 255, 0), fg="black")
btn_yellow.pack(pady=5)
btn_pink = tk.Button(root, text="Pink Button", bg=rgb_to_hex(255, 105, 180), fg="black")
btn_pink.pack(pady=5)
btn_gray = tk.Button(root, text="Gray Button", bg=rgb_to_hex(128, 128, 128), fg="white")
btn_gray.pack(pady=5)
root.mainloop()
Output in Windows:
Three buttons appear with yellow, pink, and gray backgrounds using dynamically converted RGB values.
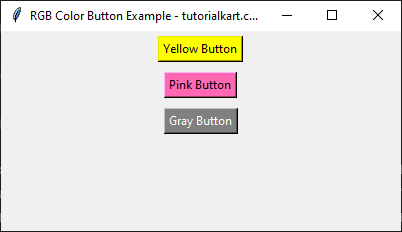
Conclusion
In this tutorial, we explored different ways to set the background and text color of a Button in Tkinter using:
- Standard color names (e.g.,
'red'
,'blue'
) - Hex color codes (e.g.,
#ff853a
) - RGB values converted to hex
By customizing button colors, you can improve the visual aesthetics of your Tkinter application.