Different Font Colors for Different Parts of a Text Widget
In Tkinter, the Text
widget allows us to display and edit multiline text. By using the tag_configure
method, we can set different font colors for different parts of the text.
The Text
widget supports tags that can be applied to specific text ranges using the text_widget.tag_add()
method. Each tag can have different properties like foreground color, background color, font, and more.
In this tutorial, we will go through detailed examples to modify text color inside a Tkinter Text Widget dynamically.
Examples
1. Setting Different Colors for Words in a Text Widget
In this example, we will create a Text
widget and apply different colors to specific words.
We use the text_widget.tag_configure()
method to define color styles and apply them to selected text ranges using text_widget.tag_add()
. The tags 'red_tag'
and 'blue_tag'
are applied to “Hello” and “World,” respectively.
main.py
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Text Widget Colors - tutorialkart.com")
root.geometry("400x200")
# Create Text widget
text_widget = tk.Text(root, font=("Arial", 14))
text_widget.pack(expand=True, fill="both")
# Insert text
text_widget.insert("1.0", "Hello World!")
# Define tag configurations for different colors
text_widget.tag_configure("red_tag", foreground="red")
text_widget.tag_configure("blue_tag", foreground="blue")
# Apply tags to specific text parts
text_widget.tag_add("red_tag", "1.0", "1.5") # "Hello" in red
text_widget.tag_add("blue_tag", "1.6", "1.11") # "World!" in blue
# Run the application
root.mainloop()
Output in Windows:
The word “Hello” is displayed in red, while “World!” appears in blue.
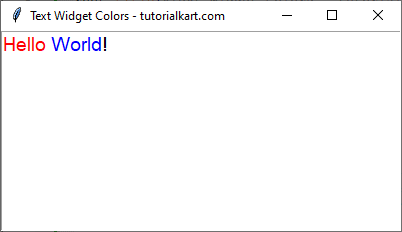
2. Highlighting Specific Words in a Paragraph in Text Widget
In this example, we will insert a paragraph into a Text
widget and highlight keywords in different colors.
We define tag_configure()
to style specific words like “Python” in green and “Tkinter” in orange. Using text_widget.tag_add()
, we locate and highlight these words dynamically.
main.py
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Text Widget Word Highlight - tutorialkart.com")
root.geometry("400x200")
# Create Text widget
text_widget = tk.Text(root, font=("Arial", 12))
text_widget.pack(expand=True, fill="both")
# Insert text
text_content = "Python is a popular programming language. Tkinter is used for GUI development in Python."
text_widget.insert("1.0", text_content)
# Define tag configurations for color highlights
text_widget.tag_configure("green_tag", foreground="green")
text_widget.tag_configure("orange_tag", foreground="orange")
# Apply tags to highlight specific words
text_widget.tag_add("green_tag", "1.0", "1.6") # "Python" in green
text_widget.tag_add("orange_tag", "1.42", "1.49") # "Tkinter" in orange
# Run the application
root.mainloop()
Output in Windows:
The word “Python” appears in green, and “Tkinter” is displayed in orange.
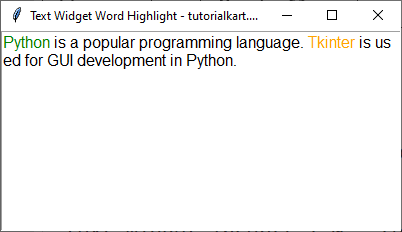
Conclusion
In this tutorial, we explored how to use the Text
widget’s tag_configure()
and tag_add()
methods to apply different font colors to specific parts of the text dynamically.