Set Font Color for Entry Widget in Tkinter Python
In Tkinter, the fg
(foreground) option of an Entry
widget allows you to set the font color of the text entered into the input field. The font color can be defined using different formats, including:
- 4-bit per color hex code (
#rgb
) - 8-bit per color hex code (
#rrggbb
) - 12-bit per color hex code (
#rrrgggbbb
) - Standard color names (
'red'
,'blue'
,'green'
, etc.)
In this tutorial, we will go through examples using the fg
option to modify the font color of a Tkinter Entry Widget.
Examples
1. Setting Font Color for Entry Widget Using 4-bit Per Color Hex Code
In this example, we will create a Tkinter Entry widget and set the text color using a 4-bit per color hex code (#rgb
format).
import tkinter as tk
root = tk.Tk()
root.title("Entry Font Color Example - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget with a red font color using a 4-bit hex code
entry = tk.Entry(root, fg="#f00", font=("Arial", 14))
entry.pack(pady=20)
root.mainloop()
Output in Windows:
A text entry field appears with red-colored text.
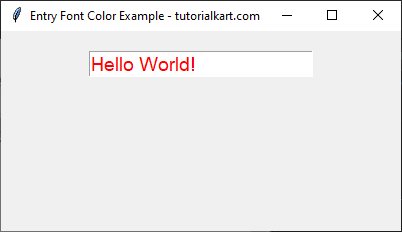
2. Setting Font Color for Entry Widget Using 8-bit Per Color Hex Code
In this example, we will set the text color using an 8-bit per color hex code (#rrggbb
format).
import tkinter as tk
root = tk.Tk()
root.title("Entry Font Color Example - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget with an orange font color using an 8-bit hex code
entry = tk.Entry(root, fg="#ff853a", font=("Arial", 14))
entry.pack(pady=20)
root.mainloop()
Output in Windows:
A text entry field appears with orange-colored text.
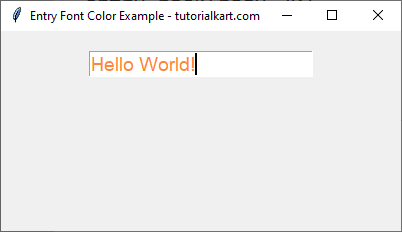
3. Setting Font Color for Entry Widget Using 12-bit Per Color Hex Code
In this example, we will set the text color using a 12-bit per color hex code (#rrrgggbbb
format).
import tkinter as tk
root = tk.Tk()
root.title("Entry Font Color Example - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget with a custom shade using a 12-bit hex code
entry = tk.Entry(root, fg="#001234bba", font=("Arial", 14))
entry.pack(pady=20)
root.mainloop()
Output in Windows:
A text entry field appears with a custom shade of text color.
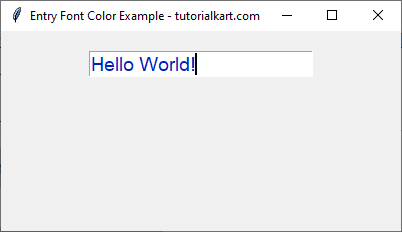
4. Setting Font Color for Entry Widget Using Standard Color Names
In this example, we will set the text color using a standard color name like 'red'
, 'blue'
, and 'green'
.
import tkinter as tk
root = tk.Tk()
root.title("Entry Font Color Example - tutorialkart.com")
root.geometry("400x200")
# Create Entry widgets with different text colors
entry_red = tk.Entry(root, fg="red", font=("Arial", 14))
entry_red.pack(pady=5)
entry_blue = tk.Entry(root, fg="blue", font=("Arial", 14))
entry_blue.pack(pady=5)
entry_green = tk.Entry(root, fg="green", font=("Arial", 14))
entry_green.pack(pady=5)
root.mainloop()
Output in Windows:
Three text entry fields appear with red, blue, and green-colored text.
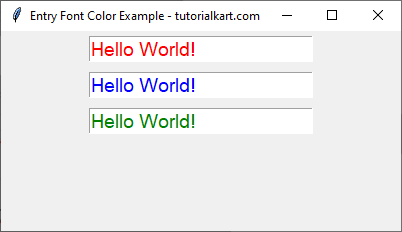
Conclusion
In this tutorial, we explored how to use the fg
option in Tkinter’s Entry widget to control the font color of the text input. The font color can be set using:
- 4-bit per color hex codes (
#rgb
) - 8-bit per color hex codes (
#rrggbb
) - 12-bit per color hex codes (
#rrrgggbbb
) - Standard color names (
'red'
,'blue'
, etc.)