Font Color for Text Widget in Tkinter Python
In Tkinter, the Text
widget is used to create multi-line text areas, and you can customize the font color of the text using the fg
(foreground) option. This allows you to enhance the appearance of text-based inputs and outputs in your GUI application.
The text color can be specified using:
- Standard color names (e.g.,
"red"
,"blue"
,"green"
) - Hexadecimal values (e.g.,
"#ff0000"
for red,"#00ff00"
for green) - RGB triplets (Not directly supported but can be converted to hex)
In this tutorial, we will go through detailed examples using the fg
option to modify the text color in a Tkinter Text Widget.
Examples
1. Setting Text Color in a Tkinter Text Widget
In this example, we will create a Tkinter Text widget and set the font color of its text using the fg
property. The text will be displayed in red.
We begin by creating the main window and setting its size using root.geometry("400x200")
. Next, we define a Text
widget and specify fg="red"
to make the text color red. We then insert some default text using text_widget.insert(tk.END, "Welcome to Tkinter!")
. Finally, the widget is packed and the main loop is started.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Text Widget Font Color - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget with red text color
text_widget = tk.Text(root, fg="red", font=("Arial", 12))
text_widget.pack(pady=10)
# Insert some text
text_widget.insert(tk.END, "Welcome to Tkinter!")
# Run the application
root.mainloop()
Output in Windows:
A multi-line text widget appears with the message “Welcome to Tkinter!” displayed in red.
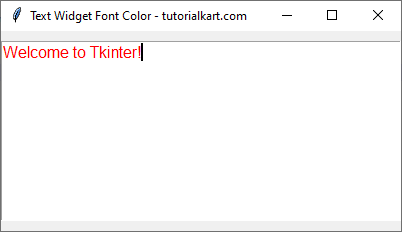
2. Setting Different Font Colors for Different Parts of Text Widget
In this example, we will modify a Tkinter Text widget to apply different colors to different parts of the text. This is done using the tag_configure
method.
We start by creating the main window and setting its size with root.geometry("400x200")
. The Text
widget is created and placed inside the window. Using text_widget.tag_configure()
, we define two tags: "color_red"
for red text and "color_blue"
for blue text. Finally, we insert two text portions and apply the respective tags using text_widget.tag_add()
.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Multi-Color Text in Tkinter - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, font=("Arial", 12))
text_widget.pack(pady=10)
# Configure tags for different colors
text_widget.tag_configure("color_red", foreground="red")
text_widget.tag_configure("color_blue", foreground="blue")
# Insert text and apply colors
text_widget.insert(tk.END, "This is red text.\n", "color_red")
text_widget.insert(tk.END, "This is blue text.", "color_blue")
# Run the application
root.mainloop()
Output in Windows:
The text widget displays two lines of text, with the first line in red and the second line in blue.
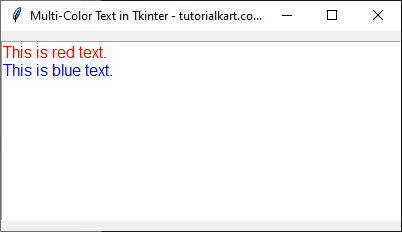
Conclusion
In this tutorial, we explored how to use the fg
option and tag_configure
in Tkinter’s Text widget to set font color. We covered:
- Changing the text color of the entire Text widget using the
fg
option. - Using
tag_configure
to apply different colors to different text portions.