How to Set Font Family for Label in Tkinter Python
In Tkinter, the font
option of a Label widget allows you to customize the font family, size, and style of the text. The font family specifies the typeface to be used for the text display.
To set the font family in a Tkinter Label, use the font
parameter while creating the Label. The format for specifying a font is:
tk.Label(root, text="Sample Text", font=("Font Family", Size, "Style"))
In this tutorial, we will go through multiple examples using the font
option to modify the font family of a Tkinter Label.
Examples
1. Setting Font Family to Arial
In this example, we will set the font family of a Label to Arial
.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Font Family Example - tutorialkart.com")
root.geometry("400x200")
# Create a Label with Arial font
label = tk.Label(root, text="Hello, Tkinter!", font=("Arial", 16))
label.pack(pady=20)
root.mainloop()
Output in Windows:
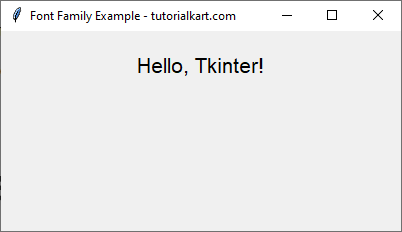
2. Setting Font Family to Times New Roman
Here, we set the font family of a Label to Times New Roman
and increase the size.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Font Family Example - tutorialkart.com")
root.geometry("400x200")
# Create a Label with Times New Roman font
label = tk.Label(root, text="Tkinter Label Example", font=("Times New Roman", 18))
label.pack(pady=20)
root.mainloop()
Output in Windows:
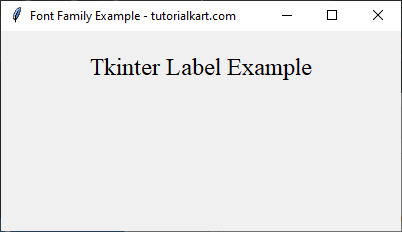
3. Setting Font Family to Courier with Bold Style
In this example, we use the Courier
font family and apply the bold
style.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Font Family Example - tutorialkart.com")
root.geometry("400x200")
# Create a Label with Courier font and bold style
label = tk.Label(root, text="Bold Courier Font", font=("Courier", 14, "bold"))
label.pack(pady=20)
root.mainloop()
Output in Windows:
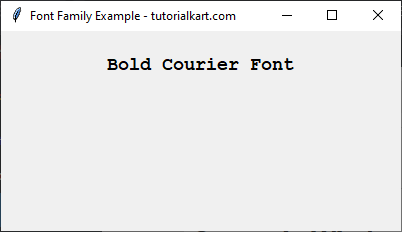
4. Using a Custom Font Family
In this example, we use the Comic Sans MS
font family.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Font Family Example - tutorialkart.com")
root.geometry("400x200")
# Create a Label with Comic Sans MS font
label = tk.Label(root, text="Custom Font Example", font=("Comic Sans MS", 16, "italic"))
label.pack(pady=20)
root.mainloop()
Output in Windows:
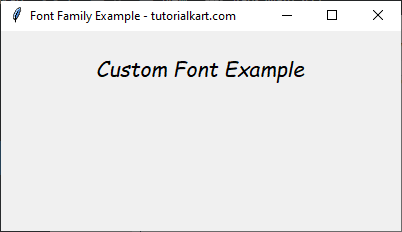
Conclusion
In this tutorial, we explored how to use the font
option in Tkinter’s Label widget to change the font family. The font can be set using a tuple containing:
- Font Family (e.g.,
"Arial"
,"Times New Roman"
,"Courier"
) - Font Size (e.g.,
16
,18
) - Optional Styles (
"bold"
,"italic"
, etc.)
By customizing the font family, you can enhance the visual appearance of labels in your Tkinter application.