Font Family for Text Widget
In Tkinter, the Text
widget allows users to enter and edit multiple lines of text. You can customize the font family of the text using the font
parameter. The font family can be specified as a tuple in the format ("Font-Family", Size, "Style")
.
In this tutorial, we will go through examples using the font
option to modify the font family of text in a Tkinter Text Widget.
Examples
1. Setting a Custom Font Family in Text Widget
In this example, we will create a Text widget and set a custom font family to Arial
with a size of 14. The text widget allows users to type and edit text with the specified font settings.
We first initialize the Tkinter window and create a Text
widget. The font is specified using the font
parameter, where we define ("Arial", 14)
. The pack()
method is used to place the widget inside the window.
main.py
import tkinter as tk
# Create the main application window
root = tk.Tk()
root.title("Text Widget Font Example - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget with a custom font
text_widget = tk.Text(root, font=("Arial", 14))
text_widget.pack(expand=True, fill="both", padx=10, pady=10)
# Run the application
root.mainloop()
Output in Windows:
A Text widget appears with a default white background.
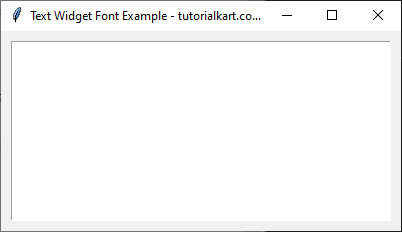
The text inside the widget is displayed in the Arial font with a size of 14.
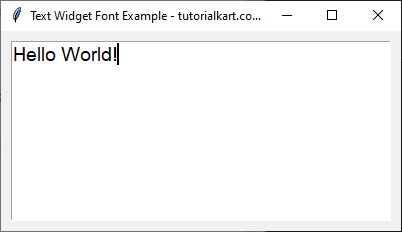
2. Using Different Font Styles in a Text Widget
In this example, we will create a Text widget and set different font families and styles to customize the appearance of the text. The first part of the text will be in Times New Roman
, and another portion will be in Courier
.
We first create the Text
widget with a default font. Then, using the tag_configure
method, we define separate font styles for different text segments. The insert
method is used to add text and apply different font styles using tag_add
.
main.py
import tkinter as tk
# Create the main application window
root = tk.Tk()
root.title("Multiple Font Styles in Text Widget - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root)
text_widget.pack(expand=True, fill="both", padx=10, pady=10)
# Define font styles
text_widget.tag_configure("times", font=("Times New Roman", 14))
text_widget.tag_configure("courier", font=("Courier", 12, "italic"))
# Insert text with different font styles
text_widget.insert("1.0", "This is Times New Roman.\n", "times")
text_widget.insert("2.0", "This is Courier Italic text.", "courier")
# Run the application
root.mainloop()
Output in Windows:
A Text widget appears with two lines of text. The first line is displayed in the Times New Roman font with a size of 14, and the second line is displayed in the Courier font with a size of 12 in italic style.
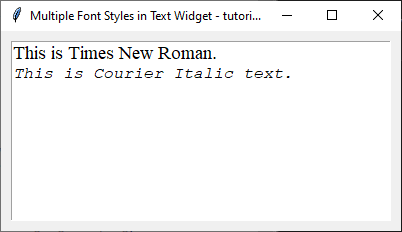
Conclusion
In this tutorial, we explored how to set the font family in a Tkinter Text widget. The font can be customized using the font
parameter while creating the Text widget or by using tag_configure
for multiple font styles.
- The font can be defined as a tuple
("Font-Family", Size, "Style")
. - Multiple font styles can be applied using
tag_configure
andtag_add
. - Font customization enhances readability and improves the visual appeal of Tkinter applications.