Set Font Size for Entry Widget in Tkinter Python
In Tkinter, the font
option of an Entry
widget allows you to set the font size of the text inside the entry field. The font size is specified as part of the font tuple, which consists of the font family, font size, and optional font style.
To set the font size for an Entry widget in Tkinter, pass the font
parameter with the desired font name and size while creating the Entry widget.
In this tutorial, we will go through examples using the font
option to modify the font size in a Tkinter Entry widget.
Examples
1. Setting Font Size in Entry Widget
In this example, we will create a Tkinter window with an Entry widget using a font size of 16.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("tutorialkart.com - Entry Font Size Example")
root.geometry("400x200")
# Create an Entry widget with a font size of 16
entry = tk.Entry(root, font=("Arial", 16))
entry.pack(pady=20)
# Run the main event loop
root.mainloop()
Output in Windows:
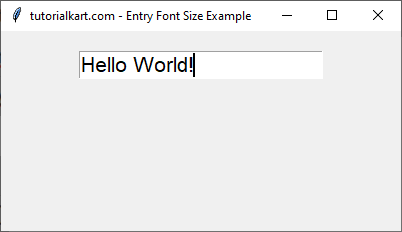
2. Setting Font Size with Bold Style for Text in Entry Widget
In this example, we set the font size to 18 and apply the bold
style.
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Entry Font Bold Example")
root.geometry("400x200")
# Create an Entry widget with bold text
entry = tk.Entry(root, font=("Arial", 18, "bold"))
entry.pack(pady=20)
root.mainloop()
Output in Windows:
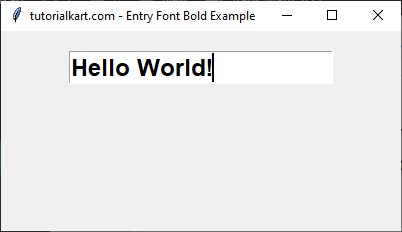
3. Setting Font Size with Italic Style for Text in Entry Widget
In this example, we set the font size to 20 with an italic style.
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Entry Font Italic Example")
root.geometry("400x200")
# Create an Entry widget with italic text
entry = tk.Entry(root, font=("Arial", 20, "italic"))
entry.pack(pady=20)
root.mainloop()
Output in Windows:
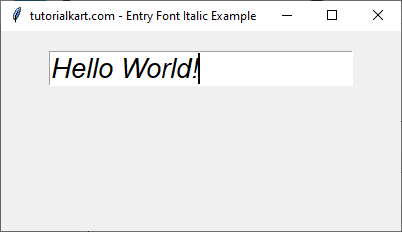
4. Setting Font Size Dynamically for Entry Widget
In this example, we create an Entry widget with a default font size and a button that changes the font size dynamically.
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Dynamic Font Size Example")
root.geometry("400x200")
# Create an Entry widget with a default font size
entry = tk.Entry(root, font=("Arial", 14))
entry.pack(pady=20)
# Function to increase font size
def increase_font():
entry.config(font=("Arial", 20))
# Create a button to increase font size
btn = tk.Button(root, text="Increase Font Size", command=increase_font)
btn.pack(pady=10)
root.mainloop()
Output in Windows:
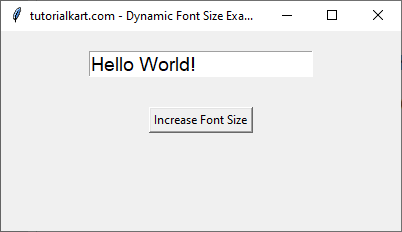
Click on Increase Font Size button.
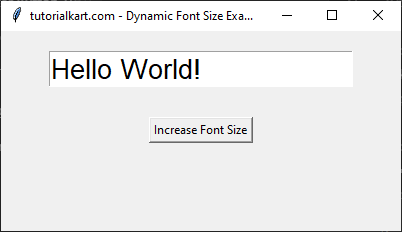
Conclusion
In this tutorial, we explored how to use the font
option in Tkinter’s Entry widget to control the font size. We learned that:
- Font size can be set using the
font
parameter in the format(font-family, size, style)
. - We can apply different styles such as
bold
anditalic
. - The font size can be dynamically changed using the
config
method.